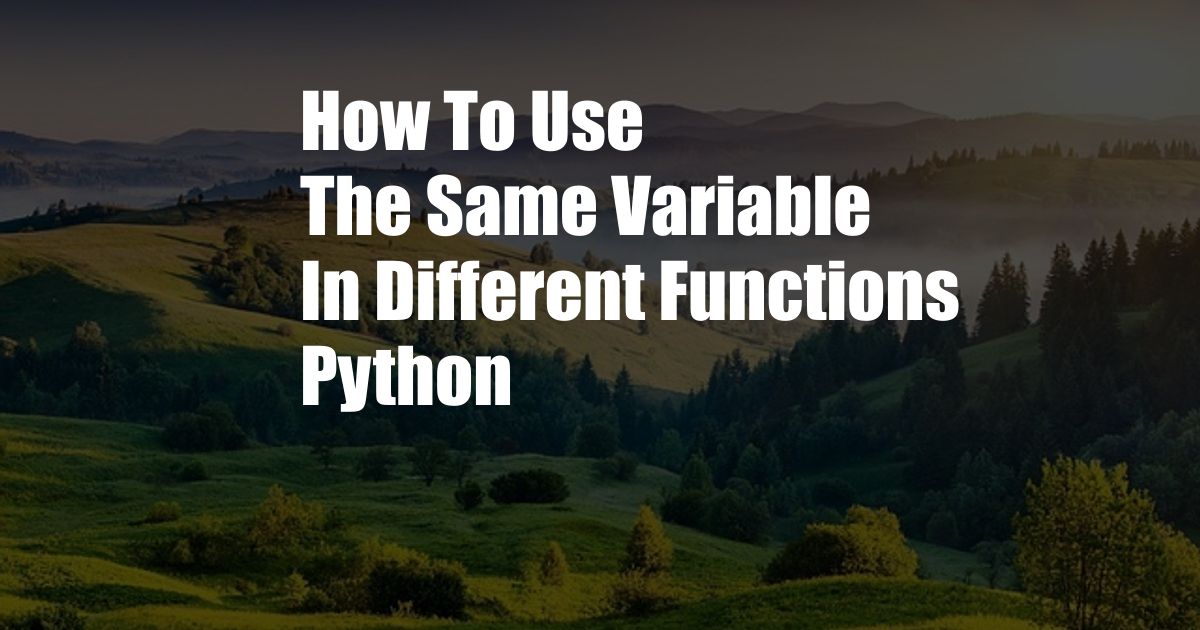
<h2>How to Use the Same Variable in Different Functions in Python</h2>
<p>In the realm of programming, variables are like precious gems, holding valuable information that helps our code function seamlessly. However, what happens when we want to access or modify the same variable from different parts of our program, particularly across multiple functions? Python, a versatile and powerful language, provides several ways to achieve this feat, offering flexibility and code reusability.</p>
<p>To embark on this exploration, let's dive into the concept of variable scope in Python.</p>
<h3>Variable Scope in Python</h3>
<p>In Python, variables have a specific scope, which determines their visibility and accessibility within a program. There are two primary scopes: local and global. Local variables are created and accessible only within the function they are defined in. Global variables, on the other hand, are declared outside any function and are accessible throughout the entire program.</p>
<h3>Using Global Variables</h3>
<p>If you need to access the same variable in multiple functions, one approach is to declare it as a global variable. This makes the variable accessible to all functions within the program. To declare a global variable, use the <code>global</code> keyword before the variable name:</p>
<pre>
global my_variable
</pre>
<p>However, it's important to use global variables judiciously. Overuse can lead to code that's difficult to understand and maintain. Additionally, modifying global variables from within functions can have unintended consequences, potentially affecting other parts of your program.</p>
<h3>Using Function Arguments</h3>
<p>A more common and preferred approach is to pass the variable as an argument to the functions that need to access it. This allows for more control and encapsulation, as the variable's scope is limited to the function call:</p>
<pre>
def my_function(my_variable):
# do something with my_variable
</pre>
<p>When calling the function, simply pass the variable as an argument:</p>
<pre>
my_variable = 10
my_function(my_variable)
</pre>
<h3>Using Return Values</h3>
<p>Another technique is to use return values. A function can return a value that can then be stored in a variable in the calling function:</p>
<pre>
def my_function():
return 10
my_variable = my_function()
</pre>
<p>Using return values provides a convenient way to share data between functions, but it can become cumbersome if multiple variables need to be returned.</p>
<h3>Using a Module</h3>
<p>For more complex scenarios where multiple functions need to share several variables, consider using a module. A module is a separate Python file that contains a collection of variables, functions, and classes. You can import the module into your program and access the variables directly:</p>
<pre>
import my_module
my_variable = my_module.my_variable
</pre>
<h3>Tips and Expert Advice</h3>
<p>In addition to the techniques mentioned above, here are some additional tips and expert advice for using variables across functions in Python:</p>
<ul>
<li><strong>Minimize global variable usage:</strong> Overuse of global variables can lead to spaghetti code and maintenance issues.</li>
<li><strong>Use descriptive variable names:</strong> Clear and concise variable names help improve code readability and understanding.</li>
<li><strong>Document your code:</strong> Adding comments to your code explains the purpose and usage of variables, making it easier for others to understand.</li>
<li><strong>Test your code thoroughly:</strong> Write unit tests to ensure that your code behaves as expected, even when variables are shared across functions.</li>
</ul>
<h3>Frequently Asked Questions (FAQs)</h3>
<ol>
<li><strong>Q: Can I access a local variable from outside the function it's defined in?</strong>
A: No, local variables are only accessible within the function they are defined in.</li>
<li><strong>Q: What's the difference between accessing a global variable vs. a function argument?</strong>
A: Accessing a global variable directly modifies the global state, while passing a variable as an argument creates a local copy within the function.</li>
<li><strong>Q: Can I modify a global variable from within a function?</strong>
A: Yes, but it's generally not recommended as it can lead to unintended consequences.</li>
</ol>
<h3>Conclusion</h3>
<p>Understanding how to use the same variable in different functions is a fundamental concept in Python programming. By leveraging global variables, function arguments, return values, and modules, you can effectively share data between functions and build more modular and reusable code.</p>
<p>Are you interested in learning more about variable scope and sharing in Python? Share your thoughts and questions in the comments below.</p>