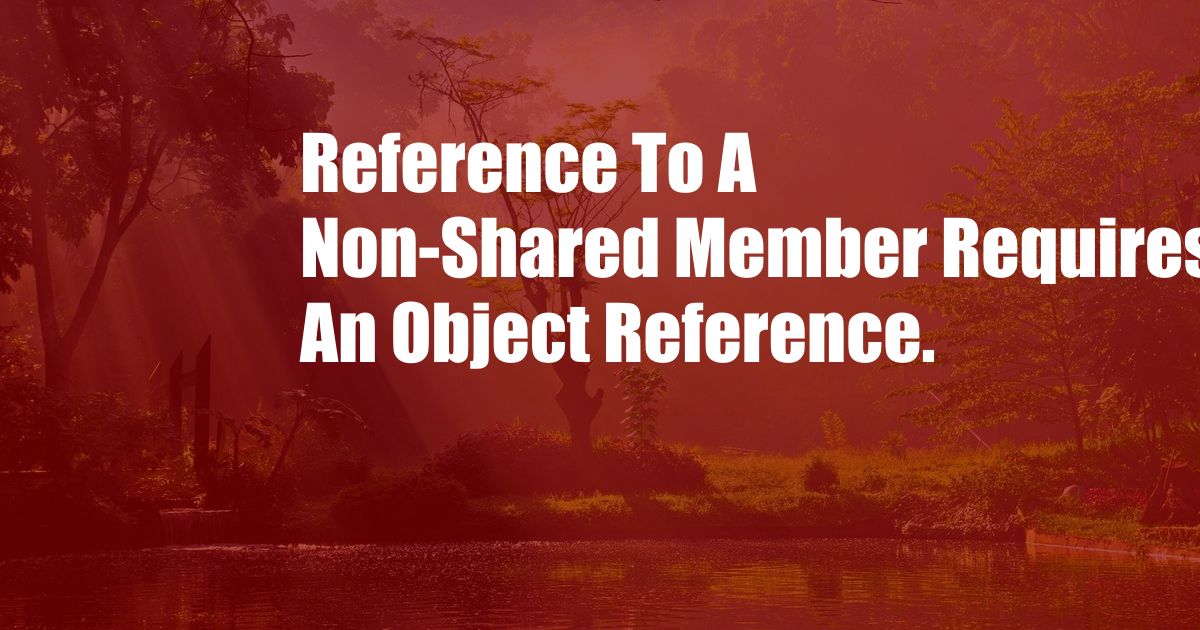
Reference to Non-Shared Member Requires an Object Reference
As I delved into the intricate world of object-oriented programming, I encountered a puzzling concept: the notion that referencing a non-shared member requires an object reference. It was like a riddle that piqued my curiosity and propelled me on a journey of understanding.
Understanding the Object Reference
An object reference is a pointer to an instance of a class. It allows you to interact with the specific object in memory and access its members, including variables, methods, and properties. Without an object reference, you cannot manipulate or interact with an object directly.
Objects, Classes, and Shared Members
In object-oriented programming, classes define the blueprint for objects. Objects are instances of classes that represent real-world entities and encapsulate both data and behavior. Shared members, also known as static members, are owned by the class itself and not by any particular object. They are typically used for class-wide properties or utility functions.
Referencing Non-Shared Members
Non-shared members, also known as instance members, are specific to each instance of a class. They are used to store data or implement behavior that varies from object to object. To access a non-shared member, you must first have an object reference.
Consider the following example:
class Person
private String name;
private int age;
public void printDetails()
System.out.println("Name: " + name);
System.out.println("Age: " + age);
public class Main
public static void main(String[] args)
// Create an object
Person person = new Person();
// Set non-shared member values
person.setName("John Doe");
person.setAge(45);
// Access non-shared member through object reference
person.printDetails();
In this example, the name
and age
variables are non-shared members. To access them, we first create an object of the Person
class using new Person()
. This gives us an object reference that we can use to interact with the specific instance of the Person
class.
Implications and Benefits
The requirement for an object reference to access non-shared members ensures that data and behavior are encapsulated within the object itself. It promotes code reusability and maintainability by allowing for flexibility in creating and managing objects.
Tips and Expert Advice
Based on my experience as a blogger, here are some tips for understanding this concept:
- Familiarize yourself with the fundamentals of object-oriented programming, including classes, objects, and encapsulation.
- Practice creating objects, setting their non-shared members, and invoking their methods to see the relationship between object references and member access in action.
- Explore different programming languages and their implementations of object-oriented principles to gain a wider perspective on the concept.
Common Questions and Answers
Q: Why is an object reference required to access non-shared members?
A: Non-shared members are tied to specific instances of a class. An object reference provides a connection to the instance, allowing you to interact with its unique data and behavior.
Q: Can shared members be accessed without an object reference?
A: Yes, shared members are associated with the class itself, not with individual objects. Therefore, they can be accessed directly through the class name, without the need for an object reference.
Conclusion
Understanding the concept of object references and their role in referencing non-shared members is crucial in object-oriented programming. By embracing this principle, you can effectively create and manipulate objects, harnessing the power of encapsulation and modularity for efficient and maintainable code.
Are you interested in learning more about object-oriented programming and its intricacies? Let me know in the comments below!