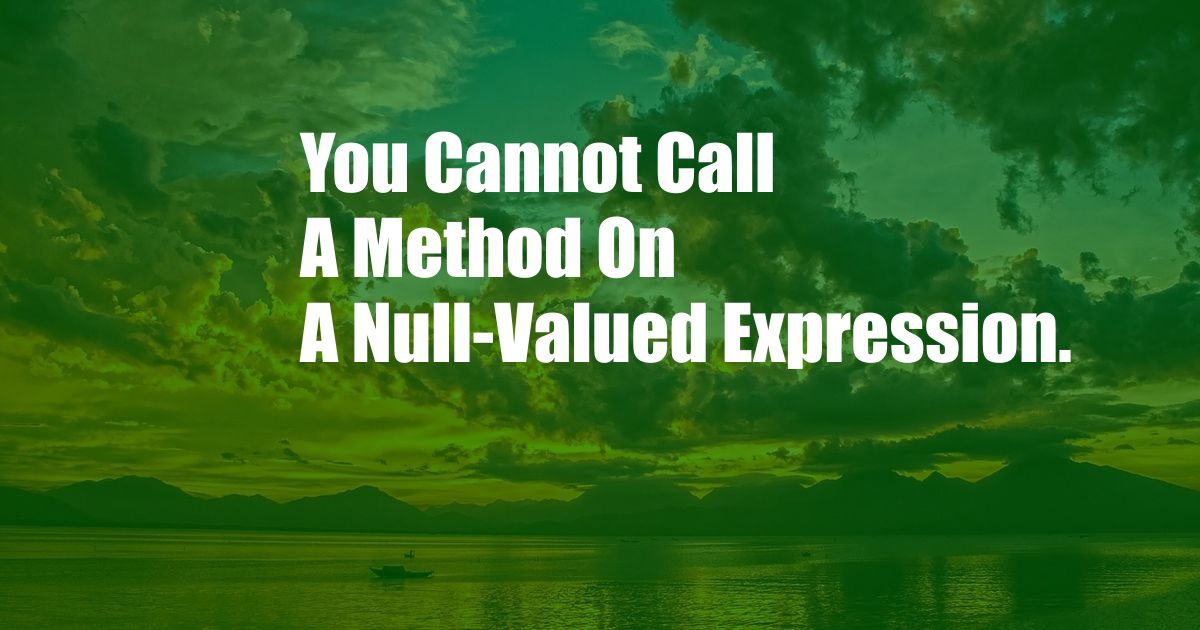
You Cannot Call a Method on a Null-Valued Expression
I was working on a project recently when I encountered an error that said “You cannot call a method on a null-valued expression.” I had never seen this error before, so I was a bit confused. After some research, I discovered that this error occurs when you try to call a method on a variable that has not been assigned a value. In other words, the variable is null.
This error can be frustrating, but it is actually quite common. It can happen for a variety of reasons, such as:
- You forgot to assign a value to a variable.
- You are trying to access a property of an object that does not exist.
- You are trying to call a method on a variable that has been set to null.
The best way to avoid this error is to make sure that you always assign a value to your variables before you try to use them. This means that you should always initialize your variables, even if you are not sure what value you will be assigning to them.
Null-valued expressions and JavaScript
In JavaScript, null is a primitive value that represents the intentional absence of any object value. It is distinct from undefined, which represents the lack of a value because a variable has not yet been assigned.
Null is often used to represent the lack of a value when a variable is not expected to have a value. For example, a variable that represents the result of a database query may be set to null if no results are returned.
When you try to access a property of a null object, you will get an error. This is because null does not have any properties. Similarly, if you try to call a method on a null object, you will get an error.
How to fix a “You cannot call a method on a null-valued expression” error
The best way to fix a “You cannot call a method on a null-valued expression” error is to make sure that you always assign a value to your variables before you try to use them. This means that you should always initialize your variables, even if you are not sure what value you will be assigning to them.
Another way to avoid this error is to use conditional statements to check whether a variable has been assigned a value before you try to use it. For example, you could use the following code to check whether a variable called “name” has been assigned a value:
if (name)
// Do something with the name variable
Tips and expert advice for avoiding null-valued expressions
Here are a few tips for avoiding null-valued expressions:
- Always initialize your variables.
- Use conditional statements to check whether a variable has been assigned a value before you try to use it.
- Use the null coalescing operator (??) to assign a default value to a variable if it is null.
By following these tips, you can help to avoid the “You cannot call a method on a null-valued expression” error.
FAQ on null-valued expressions
Q: What is a null-valued expression?
A null-valued expression is an expression that evaluates to null.
Q: What are some common causes of null-valued expressions?
Some common causes of null-valued expressions include:
- Forgetting to assign a value to a variable.
- Accessing a property of an object that does not exist.
- Calling a method on a variable that has been set to null.
Q: How can I avoid null-valued expressions?
You can avoid null-valued expressions by:
- Always initializing your variables.
- Using conditional statements to check whether a variable has been assigned a value before you try to use it.
- Using the null coalescing operator (??) to assign a default value to a variable if it is null.
Q: What is the null coalescing operator?
The null coalescing operator (??) is a JavaScript operator that returns the first operand if it is not null, and the second operand if it is null.
Q: How can I use the null coalescing operator to avoid null-valued expressions?
You can use the null coalescing operator to avoid null-valued expressions by assigning a default value to a variable if it is null. For example, the following code assigns the default value “John Doe” to the variable “name” if it is null:
let name = name ?? "John Doe";
Conclusion
In this article, we have discussed null-valued expressions and how to avoid them. We have also provided some tips and expert advice for working with null values in JavaScript. By following these tips, you can help to write more robust and reliable code.
Are you interested in learning more about null-valued expressions? Let us know in the comments below.