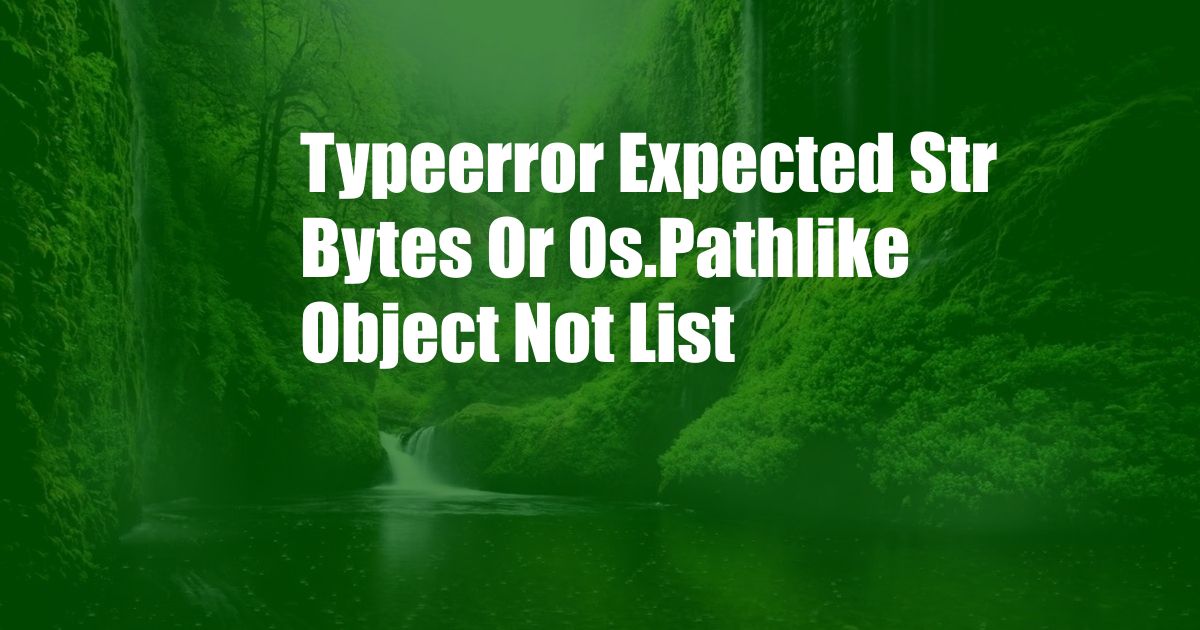
TypeError: Expected str, bytes, or os.PathLike object, not list
Have you ever encountered a frustrating error message like “TypeError: Expected str, bytes, or os.PathLike object, not list”? If you’re working with Python and dealing with file paths or data manipulation, this error can be quite common. Let’s dive into the depths of this error and explore ways to resolve it effectively.
This error typically occurs when you’re attempting an operation that expects a string, a byte-like object, or a path-like object (such as a file path). However, instead of these expected types, you’re providing a list, which is not compatible with the operation.
The Culprit: Incompatible Data Types
To understand the root cause of this error, it’s crucial to grasp the concept of data types in Python. Data types define the nature and format of data stored in variables. When you attempt an operation involving different data types, Python checks for compatibility. If the data types mismatch, as in this case where a list is supplied instead of a string or path, the operation fails, resulting in the “TypeError: Expected str, bytes, or os.PathLike object, not list” error.
Let’s illustrate this with an example. Suppose you have a function that reads data from a file. This function expects a file path as input. If you mistakenly pass a list of file paths instead of a single path, Python will raise the error because a list is not a valid file path. The function is expecting a string, representing the file path, but instead, it’s receiving a list of strings.
Resolving the Issue: Matching Expectations
Resolving this error is straightforward once you understand the mismatch in data types. The key is to ensure that the data you provide matches the type expected by the operation. In most cases, the error message provides clues about the expected data type. In this case, the message explicitly states that a string, byte-like object, or path-like object is required.
To rectify the issue, you need to convert your data to the correct type. For instance, if you have a list of file paths and want to pass them to a function expecting a single file path, you can use the join()
function to concatenate the paths into a single string. Alternatively, you can modify the function to accept a list of paths as input, adapting it to your specific use case.
Expert Advice: Avoiding the Pitfalls
To prevent encountering this error in the future, consider these expert tips:
- Understand data types and their compatibility: Familiarize yourself with the different data types in Python and their compatibility with various operations.
- Review function documentation: Before using a function, carefully read its documentation to understand its input and output data types.
- Test your assumptions: Don’t assume that data types will always match your expectations. Use Python’s
type()
function to verify data types during development. - Use type annotations: Python 3.6 and above support type annotations that can help identify potential data type mismatches during code inspection.
FAQs: Unraveling Common Queries
- Q: Why do I get this error when trying to open a file?
A: Ensure that you’re providing a valid file path as a string. If you’re using a list of paths, convert it to a single string using the
join()
function. - Q: How can I fix this error when dealing with file I/O?
A: Verify that you’re using the correct data type for file paths. Convert lists of paths to strings or modify your code to handle lists of paths explicitly.
- Q: What are the possible causes of this error?
A: The error typically occurs when you pass a list instead of a string, byte-like object, or path-like object to an operation that expects one of these types.
Conclusion
The “TypeError: Expected str, bytes, or os.PathLike object, not list” error is a common pitfall for Python developers working with file paths or data manipulation. By understanding the underlying cause of the error and implementing the recommended solutions, you can effectively resolve it and enhance your Python programming skills. Remember, careful attention to data types and function requirements goes a long way in preventing such errors and ensuring smooth execution of your Python code.
Are you interested in learning more about data types and error handling in Python? Join the discussion on our forums or explore our extensive knowledge base for additional insights.