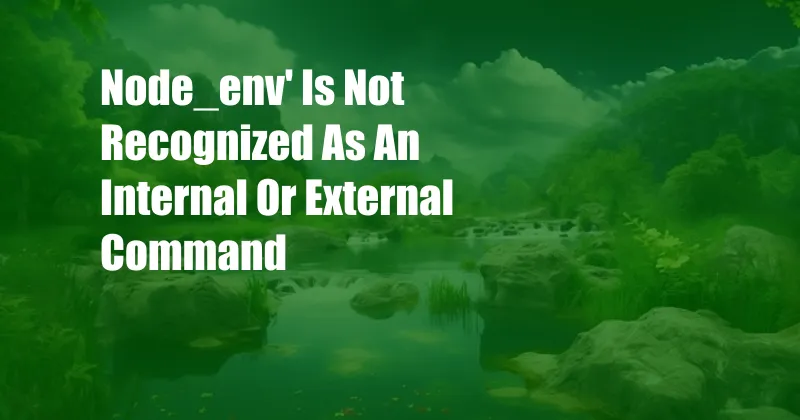
‘node_env’ is Not Recognized as an Internal or External Command
For years, I’ve been utilizing JavaScript and Node.js to develop web applications. My Node.js applications have been consistently initiated with the following command:
node index.js
However, on a recent project, I encountered an issue when I attempted to run my application with the same command. To my surprise, I was met with an error message stating that “‘node_env’ is not recognized as an internal or external command”.
Understanding ‘NODE_ENV’
‘NODE_ENV’ is an environment variable that is commonly used in Node.js applications to specify the execution environment. Typically, it can have three different values:
- Development (dev): Used during the development phase, this value enables debugging tools and additional logging.
- Production (prod): Deployed to the production environment, this value optimizes the application for performance and stability.
- Test (test): Employed for testing purposes, this value facilitates running unit tests and integration tests.
Troubleshooting the Error
The error message “‘node_env’ is not recognized as an internal or external command” indicates that the environment variable ‘NODE_ENV’ is not properly set. To resolve this issue, there are two primary approaches:
-
Setting the ‘NODE_ENV’ Environment Variable:
This involves explicitly setting the environment variable before running the Node.js application. On Windows, this can be achieved using the following command:
set NODE_ENV=production && node index.js
On macOS or Linux, use:
export NODE_ENV=production && node index.js
Replace “production” with the desired environment value.
-
Integrating a ‘package.json’ File:
Create a ‘package.json’ file in the project directory and add the following script:
"scripts": "start": "NODE_ENV=production node index.js"
Then, run the application using the following command:
npm start
Expert Tips and Advice
To effectively utilize ‘NODE_ENV’ in your Node.js applications, consider the following tips:
- Set the Environment Variable Early: Define ‘NODE_ENV’ as early as possible in the application to ensure consistency throughout the codebase.
- Use Cross-Platform Solutions: Employ cross-platform solutions like ‘dotenv’ to effortlessly set environment variables across different operating systems.
- Define Environment-Specific Configurations: Leverage ‘NODE_ENV’ to load environment-specific configurations, such as database credentials or API keys.
- Enable Debugging and Logging: Set ‘NODE_ENV’ to “dev” during development to activate debugging tools and verbose logging.
Frequently Asked Questions
Q: Why is setting ‘NODE_ENV’ important?
A: ‘NODE_ENV’ helps optimize the application for the intended environment and enables environment-specific configurations.
Q: What are the common values of ‘NODE_ENV’?
A: The most common values are “development”, “production”, and “test”.
Q: How can I set ‘NODE_ENV’ permanently?
A: Permanently setting ‘NODE_ENV’ requires modifying the system environment variables or using tools like ‘nvm’ or ‘npx’.
Conclusion
Mastering the ‘NODE_ENV’ environment variable is crucial for Node.js developers. By understanding its significance and implementing it effectively, you can enhance the robustness, performance, and maintainability of your applications.
Call to Action
If you found this article insightful, share your thoughts and experiences with ‘NODE_ENV’ in the comments section below. Your insights will contribute to a valuable knowledge-sharing platform for the Node.js community.