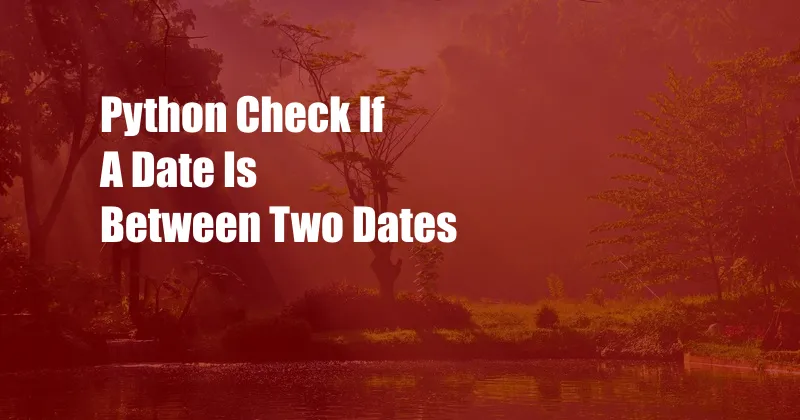
Checking Date Ranges with Python: A Comprehensive Guide
As a data analyst, I’ve often encountered the need to determine if a date falls within a specific range. In the past, I struggled with this task, but thanks to Python’s datetime library, it’s now a breeze. In this blog post, I’ll guide you through the intricacies of checking date ranges, empowering you with the knowledge to tackle this common programming challenge.
Before diving into the technical details, let’s delve into the significance of date range checks. Imagine you’re managing a hotel reservation system. Checking if a booking date falls within the available period is crucial to avoid double bookings and ensure a seamless guest experience. Similarly, in finance, verifying that transactions occur within permissible date limits is vital for compliance and fraud prevention.
Understanding Date Ranges
A date range represents a period of time between two dates. In Python, we can create date ranges using the dateutil library. The following code demonstrates how:
from dateutil.rrule import rrule, DAILY
start_date = datetime(2023, 5, 1)
end_date = datetime(2023, 5, 31)
date_range = rrule(DAILY, dtstart=start_date, until=end_date)
This code creates a date range from May 1st, 2023, to May 31st, 2023. The rrule function generates a sequence of dates based on the provided rule. In this case, the rule is DAILY, indicating that we want all the dates within the specified range.
Checking if a Date is Within a Range
Once we have a date range, we can check if a specific date falls within it. Python provides several methods to accomplish this:
1. Datetime Comparison: We can simply compare the date to the start and end dates of the range. If the date is greater than or equal to the start date and less than or equal to the end date, it’s within the range.
if date >= start_date and date <= end_date:
print("Date is within the range")
2. In Range Function: The dateutil library offers an inrange function that checks if a date is within a date range. It returns a boolean value.
from dateutil.relativedelta import relativedelta
date_range = (start_date, end_date)
if date in relativedelta.relativedelta(date_range):
print("Date is within the range")
Latest Trends in Date Range Checking
The advent of big data and real-time analytics has fueled the need for efficient date range checking algorithms. Recent advancements include:
1. Interval Trees: Interval trees are data structures optimized for storing and searching date ranges. They support efficient range queries, enabling lightning-fast date range checks in large datasets.
2. GPU-Accelerated Algorithms: Graphics processing units (GPUs) offer parallel processing capabilities that can significantly speed up date range checks in massive datasets. Researchers are exploring algorithms leveraging GPUs to achieve real-time performance.
Tips and Expert Advice
To enhance your understanding of date range checking, consider these expert tips:
1. Handle Boundary Conditions: Ensure your code correctly handles cases where the date is exactly equal to the start or end date of the range. Use >= and <= comparisons in your conditions.
2. Use Libraries: Leverage the power of libraries like dateutil, which provides a comprehensive suite of functions for date manipulation and range checking. It simplifies your code and improves accuracy.
FAQ on Date Range Checking
Q: Can I check if a date range is empty?
A: Yes, you can use the isempty function from the dateutil.rrule module to determine if a date range contains no dates.
Q: How do I exclude specific dates from a date range?
A: You can use the exdate parameter of the rrule function to exclude specific dates from a date range. Pass a list of excluded dates to this parameter.
Conclusion
Mastering the art of date range checking in Python empowers you to address various real-world problems effectively. From reservation systems to financial compliance, this skill is essential for ensuring accurate and reliable date processing. By understanding the concepts, implementing these techniques, and keeping up with the latest trends, you can become a proficient Python developer.
If you have found this guide helpful, please don’t hesitate to explore other resources on our website. We provide a wealth of information on Python programming and other topics to empower you in your coding journey.