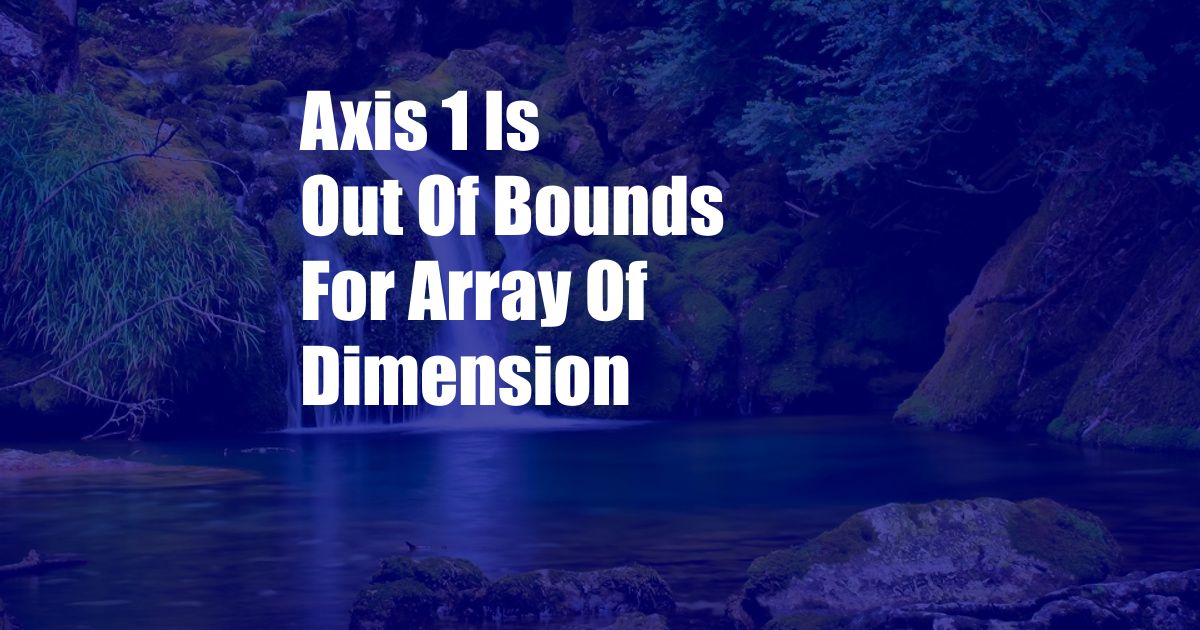
Axis 1 is Out of Bounds for Array of Dimension 1
Have you ever encountered the frustrating error message “Axis 1 is out of bounds for array of dimension 1” while working with arrays in Python? It can be particularly baffling if you’re sure you’ve defined the array correctly and provided valid indices.
To understand this error, let’s delve into the concept of arrays and their dimensions. An array is a data structure that stores a collection of elements of the same type. In Python, arrays are implemented using the NumPy library, which supports multidimensional arrays, allowing you to represent data in tabular or matrix form.
Understanding Array Dimensions
Each dimension of an array represents a level of nesting. A one-dimensional array is a simple list of elements, while a two-dimensional array can be visualized as a table with rows and columns. Higher-dimensional arrays extend this concept to more levels of nesting.
When you access an element in an array, you specify the index for each dimension. For example, in a two-dimensional array, you would provide two indices, one for the row and one for the column. The error “Axis 1 is out of bounds for array of dimension 1” occurs when you attempt to access an element using an invalid index for a specific dimension.
Identifying the Cause of the Error
There are two common reasons for this error:
- Incorrect Index Range: You may have used an index that is outside the valid range for the specified dimension. For example, if you have a one-dimensional array with 10 elements, you can only access indices from 0 to 9. Trying to access index 10 or higher would result in the error.
- Wrong Dimensionality: You may be attempting to access an element in a dimension that is not defined for the array. For instance, if you have a one-dimensional array, you cannot access elements using two indices. This error occurs because the array is not defined with that level of dimensionality.
Tips for Avoiding the Error
To avoid encountering this error, follow these tips:
- Verify Array Dimensions: Before accessing an element, check the shape of the array using the
shape
attribute. This will give you the number of dimensions and the size of each dimension. - Use Consistent Indexing: Ensure that you’re using the correct number of indices for the dimensionality of the array. For example, a two-dimensional array requires two indices, while a three-dimensional array requires three indices.
- Handle Boundary Conditions: When accessing the last element of a dimension, consider using the
len
function to determine the valid index range. This ensures that you don’t exceed the bounds of the array.
FAQ on Axis 1 Out of Bounds Error
Q: What is the difference between a one-dimensional and a two-dimensional array?
A: A one-dimensional array is a simple list of elements, while a two-dimensional array has rows and columns, allowing for tabular data representation.
Q: How can I check the dimensionality of an array?
A: Use the shape
attribute of the array to get the number of dimensions and the size of each dimension.
Q: What is the cause of the “Axis 1 is out of bounds” error?
A: This error occurs when you try to access an element in an invalid index range or a non-existent dimension.
Conclusion
Understanding the concept of array dimensions and using the correct indexing technique can help you avoid the “Axis 1 is out of bounds for array of dimension 1” error. By following the tips and guidelines provided, you can work effectively with multidimensional arrays in Python.
Would you like to learn more about arrays and their dimensions? Share your questions or insights in the comments below!