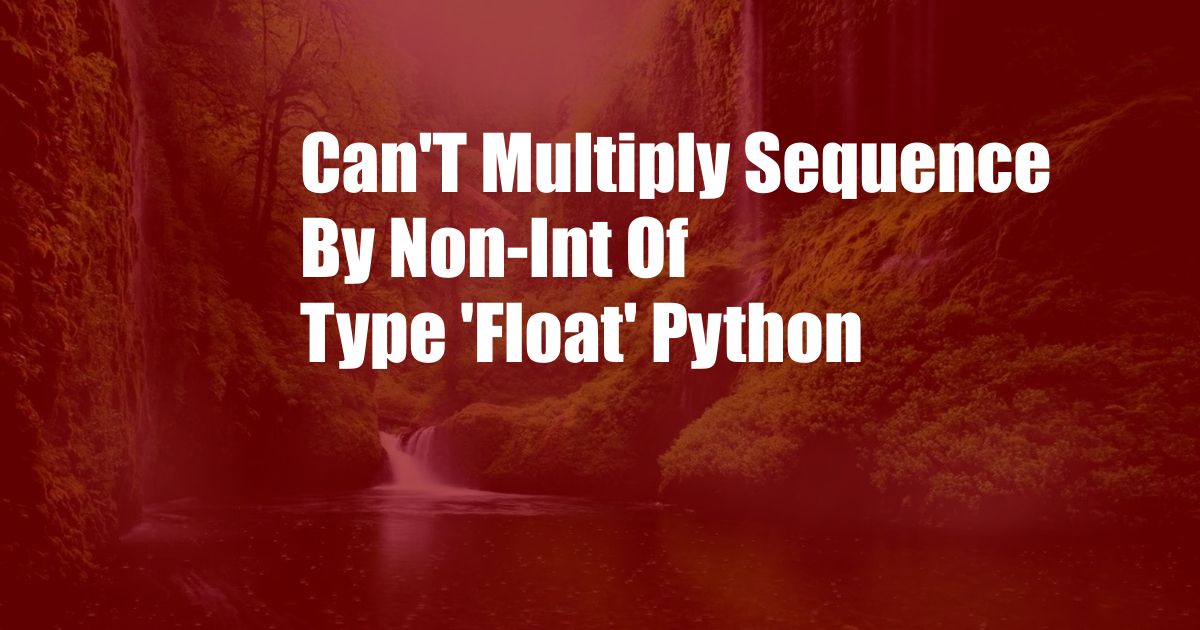
Can’t multiply sequence by non-int of type ‘float’ Python
Have you ever encountered the perplexing error message “can’t multiply sequence by non-int of type ‘float’” while working with Python? This error arises when attempting to multiply a sequence, such as a list or tuple, by a non-integer value, typically a floating-point number (float). Understanding the root cause of this error is crucial for debugging and maintaining code integrity.
To comprehend the error, it’s essential to grasp the fundamental principles of Python’s type system. In Python, data types define the characteristics and operations that can be performed on variables. Numeric types, including integers and floats, have distinct properties and limitations. Integers represent whole numbers (e.g., 1, 5, -10), while floats represent fractional or decimal numbers (e.g., 3.14, 0.5, -2.71).
Multiplication Operator (*) in Python
The multiplication operator (*) in Python performs different operations depending on the operands’ data types. When multiplying two integers, it returns the product of those integers. However, when multiplying a sequence (such as a list or tuple) by a non-integer value (e.g., a float), Python raises the “can’t multiply sequence by non-int of type ‘float’” error.
To understand why this error occurs, consider the following scenario: you have a list of numbers [1, 2, 3] and want to multiply each element by a scaling factor of 2.0. The intuitive approach would be to use the following code:
numbers = [1, 2, 3] scaling_factor = 2.0result = numbers * scaling_factor
However, this code will trigger the “can’t multiply sequence by non-int of type ‘float’” error. The reason is that Python interprets the multiplication operator (*) differently when working with sequences. In this case, the operator performs element-wise multiplication, which is only defined for sequences of the same length and element type.
Solution to the Error
To resolve the error, you need to modify the code to perform the multiplication operation correctly. Since you want to multiply each element in the list by the scaling factor, you can use a list comprehension or a for loop:
# Option 1: List comprehension numbers = [1, 2, 3] scaling_factor = 2.0result = [x * scaling_factor for x in numbers] # Option 2: For loop numbers = [1, 2, 3] scaling_factor = 2.0 result = [] for x in numbers: result.append(x * scaling_factor)
Both of these approaches will produce the desired result: [2.0, 4.0, 6.0]. By explicitly looping over the elements in the list and performing the multiplication operation individually, you can avoid the error and achieve the desired result.
Conclusion
Understanding the “can’t multiply sequence by non-int of type ‘float’” error is critical for writing error-free Python code. It stems from the distinction between element-wise operations and scalar multiplication in Python. By modifying the code to perform the multiplication operation correctly using list comprehensions or loops, you can effectively handle sequences of numeric data and avoid this common error.
If you encounter this error in the future, remember to check the data types of the operands involved and ensure that you are performing the intended operation. By following the guidelines outlined in this article, you can resolve the error efficiently and maintain the integrity of your Python code.
Are you interested in learning more about Python?
Check out our comprehensive Python Tutorial for Beginners, where you’ll find everything you need to get started with this versatile programming language. From basic concepts to advanced techniques, this tutorial will guide you through the ins and outs of Python, empowering you to build robust and efficient applications.
FAQs
Q: What causes the “can’t multiply sequence by non-int of type ‘float’” error in Python?
A: This error occurs when attempting to multiply a sequence (e.g., a list or tuple) by a non-integer value (e.g., a float) in Python.
Q: How can I resolve the “can’t multiply sequence by non-int of type ‘float’” error?
A: To resolve this error, perform the multiplication operation element-wise using list comprehensions or loops.
Q: What is element-wise multiplication in Python?
A: Element-wise multiplication in Python refers to performing multiplication operations between corresponding elements of sequences. It is only defined for sequences of the same length and element type.