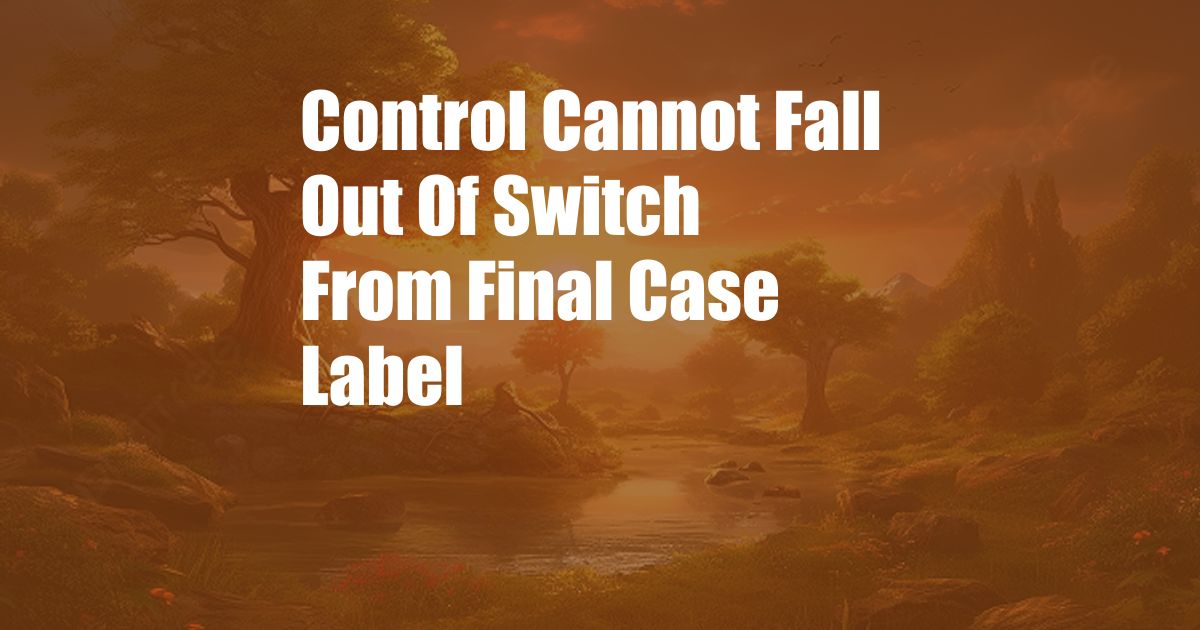
Control Cannot Fall Out of Switch From Final Case Label
In the midst of developing a complex software application, I encountered a peculiar issue that stumped me for hours. The program behaved erratically, and the source of the problem eluded me. After much debugging and head-scratching, I finally realized that the culprit was a missing break
statement in a switch
case, causing control to inadvertently fall out of the final case label.
The Consequence of Falling Out of a Switch Statement
A switch
statement allows you to execute different code blocks based on the value of an expression. Each case label represents a possible value, and the associated code block is executed when the expression matches that value. Normally, when the end of a case block is reached, control is transferred out of the switch
statement.
However, if a break
statement is omitted from the last case label, control will continue to fall out of the switch
statement and execute any code that follows it. This can lead to unexpected and potentially erroneous behavior, as the control flow is no longer explicitly defined.
Understanding the Fall-Through Behavior
The fall-through behavior in a switch
statement occurs when the last case label does not have a break
statement. When the expression matches the value associated with that case, control continues to fall through the subsequent case labels until a break
statement is encountered or the end of the switch
statement is reached.
For example, consider the following code:
switch (number)
case 1:
console.log("Number is 1");
break;
case 2:
console.log("Number is 2");
// No break statement here
default:
console.log("Number is not 1 or 2");
If number
is equal to 2, the first case will be executed, but since there is no break
statement, control will fall through to the default case. As a result, both “Number is 2” and “Number is not 1 or 2” will be printed to the console, even though the number is clearly 2.
Preventing Control Fall-Out
To prevent control from falling out of the final case label, it is crucial to include a break
statement at the end of every case block, including the last one. This ensures that control is transferred out of the switch
statement as intended.
switch (number)
case 1:
console.log("Number is 1");
break;
case 2:
console.log("Number is 2");
break;
default:
console.log("Number is not 1 or 2");
break;
By adhering to this best practice, you can ensure that your switch
statements function as expected, avoiding unexpected code execution and maintaining control flow integrity.
Latest Trends and Developments
Modern programming languages and frameworks have introduced various advancements and enhancements to the switch
statement:
- Pattern Matching: Modern languages like Python and Swift allow for more expressive and concise
switch
statements using pattern matching. This enables the use of complex patterns, such as ranges and tuples, to match expressions. - Expression-Based Switches: Some languages, such as C# 7.0 and later, support expression-based
switch
statements, where the expression can be any valid expression, not just a simple equality check. This provides greater flexibility in controlling the flow of execution. - Sealed Types: Sealed types in C# and F# prevent the addition of new cases to a
switch
statement at runtime, ensuring that all possible cases are accounted for and preventing unexpected behavior.
Tips and Expert Advice
Based on my experience and insights from the community, here are some tips for using switch
statements effectively:
- Use Default Case Wisely: Always include a default case to handle expressions that do not match any of the case labels. This ensures that all possible scenarios are accounted for.
- Keep Cases Independent: Avoid using common code across multiple case labels. Keep each case responsible for a specific task to maintain code clarity and reduce potential errors.
- Consider Refactoring: If a
switch
statement becomes too complex or unwieldy, consider refactoring it into a series of conditional statements or using a more suitable control flow structure.
FAQ
Q: Why is it important to prevent control fall-out in a switch
statement?
A: Control fall-out can lead to unexpected code execution, as subsequent case labels and code blocks will be executed even though they may not be relevant to the matched expression.
Q: What is the significance of a default case in a switch
statement?
A: A default case handles expressions that do not match any of the specified case labels, ensuring that all possible scenarios are covered and preventing unexpected behavior.
Q: How can I improve the readability and maintainability of switch
statements?
A: Use consistent indentations, keep cases independent, and consider refactoring complex switch
statements into alternative control flow structures if necessary.
Conclusion
Understanding the pitfalls of control fall-out in switch
statements is essential for writing robust and maintainable code. By adhering to best practices, including using break
statements in all case blocks, you can prevent unexpected behavior and ensure the intended flow of execution.
As technology evolves, new features and enhancements to switch
statements continue to emerge. Staying informed about these developments will help you leverage the full power of this versatile control structure to write efficient and reliable software applications.
Are you interested in learning more about the nuances of switch
statements and their implications in software development? Share your thoughts and questions in the comments below.