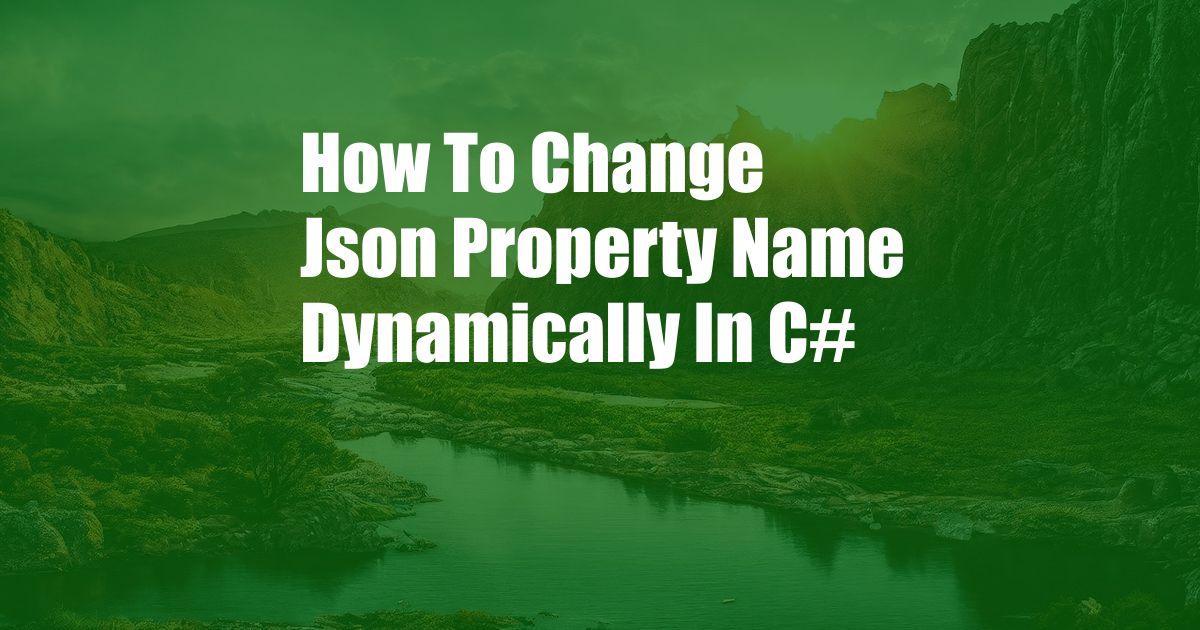
How to Dynamically Change JSON Property Names in C
In my project, I recently encountered a scenario where I needed to dynamically change the property names of a JSON object in C#. I explored different approaches and stumbled upon a versatile solution that allows for flexible property name manipulation. Let’s dive into the details of this technique.
JSON Property Manipulation
JSON (JavaScript Object Notation) is a widely used data format for data exchange. It represents data in a hierarchical structure, with properties and values represented as key-value pairs. In certain scenarios, it becomes necessary to modify the property names of a JSON object to align with specific requirements. C#, a powerful programming language, provides various mechanisms to achieve this dynamic property name manipulation.
Using Newtonsoft.Json
Newtonsoft.Json is a popular third-party library that extends the capabilities of JSON handling in C#. It offers a comprehensive set of features, including the ability to dynamically change property names. Here’s how you can leverage Newtonsoft.Json for this purpose:
- Install the Newtonsoft.Json NuGet package: This package provides the necessary classes and methods for JSON manipulation.
- Create a JSON object: Initialize a JSON object using the
JObject
class. - Modify property names using
Rename()
: TheRename()
method allows you to change the property name of an existing property. Simply specify the original property name and the new desired name. - Serialize the modified JSON object: Once the property names have been modified, you can serialize the JSON object back to a JSON string using the
ToString()
method.
Code Example
The following code snippet demonstrates how to dynamically change property names using Newtonsoft.Json:
using Newtonsoft.Json;
// Create a JSON object
JObject json = new JObject
"oldPropertyName", "Old Value" ,
"anotherOldPropertyName", "Another Old Value"
;
// Rename properties
json["oldPropertyName"].Rename("newPropertyName");
json["anotherOldPropertyName"].Rename("anotherNewPropertyName");
// Serialize the modified JSON object
string modifiedJson = json.ToString();
Latest Trends and Developments
Dynamic property name manipulation in JSON has gained significance with the advent of microservices and serverless architectures. These architectures often involve the exchange of data between different services and systems, which may adhere to different data schemas. The ability to dynamically change property names enables seamless data integration and interoperability.
Tips and Expert Advice
- Use a library like Newtonsoft.Json: It provides robust support for JSON manipulation, including dynamic property name modification.
- Consider using reflection: Reflection allows you to dynamically access and modify object properties, including JSON properties.
- Validate input and handle exceptions: Always validate the input JSON object to ensure that the property names you want to change exist. Handle any exceptions that may arise during property name manipulation.
Frequently Asked Questions
Q: Why would I need to dynamically change JSON property names?
A: You may need to change property names to align with different data schemas, support translations, or comply with changing business requirements.
Q: Can I use built-in C# features for dynamic property name manipulation?
A: While C# does not directly support dynamic property name manipulation for JSON objects, you can use reflection or third-party libraries like Newtonsoft.Json to achieve this functionality.
Conclusion
Dynamically changing JSON property names is a crucial technique for handling JSON data in C#. By leveraging libraries like Newtonsoft.Json and following best practices, you can effectively modify property names to meet specific requirements. This technique empowers developers to create flexible and interoperable data exchange solutions. Are you interested to know more about this topic?