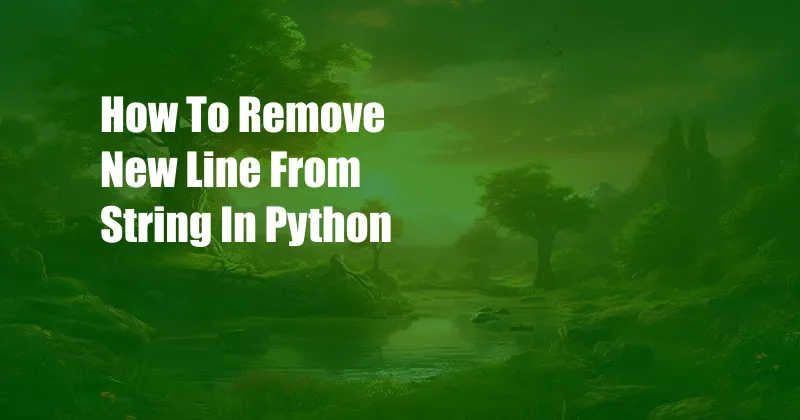
How to Remove New Line from String in Python
I remember when I was first learning Python, I was puzzled by how to remove new lines from strings. I kept getting frustrated because my code wasn’t working the way I expected it to. After some research, I finally found the answer, and I’m excited to share it with you today.
New lines can be a real pain to deal with in Python. They can cause problems when you’re trying to parse data, and they can make your code look messy. Fortunately, there are a few simple ways to remove new lines from strings in Python.
The strip() Method
The strip() method is the most common way to remove new lines from strings in Python. It takes a single argument, which is the character or characters that you want to remove from the string. By default, the strip() method removes all whitespace characters, including new lines.
>>> my_string = "Hello\nworld"
>>> my_string.strip()
'Hello world'
You can also use the strip() method to remove specific characters from a string. For example, the following code removes all instances of the letter “e” from the string:
>>> my_string = "Hello world"
>>> my_string.strip("e")
'Hllo wrold'
The replace() Method
The replace() method is another option for removing new lines from strings in Python. It takes two arguments: the character or characters that you want to remove from the string, and the character or characters that you want to replace them with.
>>> my_string = "Hello\nworld"
>>> my_string.replace("\n", "")
'Hello world'
You can also use the replace() method to remove multiple characters from a string. For example, the following code removes all instances of the letters “e” and “o” from the string:
>>> my_string = "Hello world"
>>> my_string.replace("e", "").replace("o", "")
'Hll wrld'
The split() Method
The split() method can be used to split a string into a list of substrings. By default, the split() method splits the string on whitespace characters, including new lines.
>>> my_string = "Hello\nworld"
>>> my_string.split()
['Hello', 'world']
You can also use the split() method to split the string on a specific character or characters. For example, the following code splits the string on the letter “e”:
>>> my_string = "Hello world"
>>> my_string.split("e")
['H', 'llo world']
Once you have split the string into a list of substrings, you can use the join() method to join the substrings back together into a single string. The following code joins the substrings back together into a single string with no new lines:
>>> my_string = "Hello\nworld"
>>> my_string_list = my_string.split()
>>> my_string_joined = " ".join(my_string_list)
'Hello world'
Tips and Expert Advice
Here are a few tips and expert advice for removing new lines from strings in Python:
- Use the strip() method to remove all whitespace characters, including new lines.
- Use the replace() method to remove specific characters from a string, including new lines.
- Use the split() method to split a string into a list of substrings, and then use the join() method to join the substrings back together into a single string with no new lines.
By following these tips, you can easily remove new lines from strings in Python. This will make your code more readable and easier to maintain.
FAQ
Here are some frequently asked questions about removing new lines from strings in Python:
- What is the difference between the strip() and replace() methods?
The strip() method removes all whitespace characters, including new lines, from the beginning and end of a string. The replace() method replaces all occurrences of a specified character or characters with another specified character or characters.
- What is the difference between the split() and join() methods?
The split() method splits a string into a list of substrings, and the join() method joins a list of substrings into a single string.
- How can I remove all new lines from a string?
You can remove all new lines from a string by using the strip() method to remove all whitespace characters, including new lines.
Conclusion
I hope this article has helped you learn how to remove new lines from strings in Python. If you have any questions, please feel free to leave a comment below.
Are you interested in learning more about Python? Check out our other blog posts on Python.