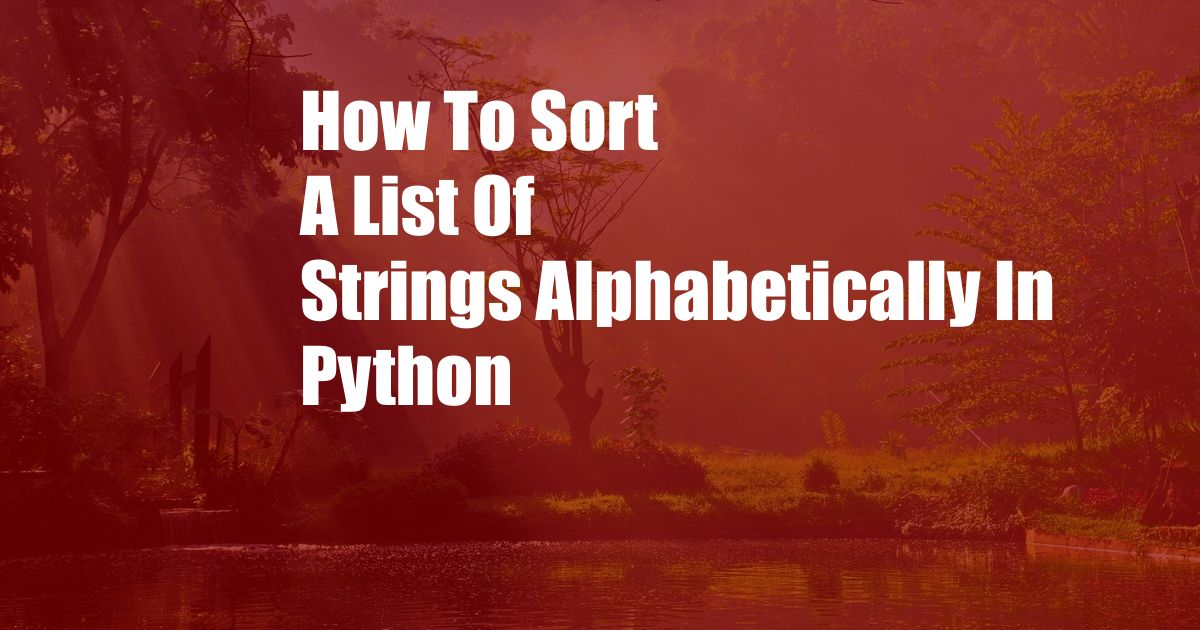
The Art of String Sorting: Unleashing Python’s Alphabetical Prowess
Standing on the bustling sidewalks of a chaotic city, you notice a towering billboard advertising the “Grand Alphabet Carnival.” A shiver of excitement runs down your spine as you imagine yourself navigating mazes of words, deciphering anagrams, and mastering the elusive art of alphabetical order.
Alphabetization in the Digital Realm
In our digital world, data reigns supreme. From vast databases to simple text files, organizing and sorting information is paramount. Among the many wonders of programming, Python shines brightly for its ability to manipulate and transform data with effortless grace. When it comes to strings—sequences of characters—Python offers a suite of tools to bring order to the chaos.
Python’s String Sorting Toolkit
Python’s string sorting arsenal is both versatile and powerful:
- str.sort(): Magically arranges the characters of a string in ascending alphabetical order.
- sorted(string): A more versatile alternative, allowing you to sort any sequence of strings, including lists and tuples.
- lambda: A powerful anonymous function that enables complex sorting criteria.
The Mechanics of Sorting
To empower you with the knowledge of these sorting techniques, let’s delve into the practicalities:
- str.sort(): With this method, you can effortlessly sort the characters within a single string. For instance, if you have the string “hello,” invoking str.sort() will transform it into “ehllo.”
- sorted(string): This versatile function grants you the ability to handle sequences of strings. Consider a list of names: [“Adam”, “Eve”, “Bob”, “Alice”]. sorted(names) will return a new list with the names arranged in alphabetical order: [“Adam”, “Alice”, “Bob”, “Eve”].
- lambda: For more intricate sorting criteria, embrace the power of lambda functions. Lambda functions allow you to define custom sorting keys based on any attribute or aspect of your strings. For example, to sort a list of file names by their extensions, you can use the following lambda function: lambda filename: filename.split(‘.’)[-1].
Latest Trends and Developments
The realm of string sorting is constantly evolving, with new techniques and algorithms emerging to cater to the ever-growing needs of data manipulation. Python’s dynamic nature ensures that it remains a pioneer in this domain, incorporating the latest advancements into its vast library.
Expert Tips and Advice
Having explored the fundamental principles of string sorting in Python, let’s delve into some valuable tips and advice from seasoned experts:
- Leverage the built-in sorting methods first: When dealing with simple sorting tasks, the built-in str.sort() and sorted() functions provide a convenient and efficient solution.
- Craft custom sorting criteria with lambda: For complex sorting scenarios, unleash the power of lambda functions to tailor your sorting logic precisely.
- Maintain code readability: As your sorting logic grows more intricate, prioritize code readability to ensure maintainability and ease of understanding.
- Test your code: Thoroughly test your sorting algorithms to ensure they handle various string sequences and edge cases flawlessly.
Frequently Asked Questions
Q: Can I sort strings in descending order?
A: Absolutely! By adding the reverse=True parameter to str.sort() or sorted(), you can reverse the sorting order, arranging strings in descending alphabetical order.
Q: How do I sort strings case-insensitively?
A: To disregard case differences during sorting, use the key=str.lower parameter in sorted(). This sorts the strings as if they were all lowercase.
Q: Can I sort a list of strings by their length?
A: Yes, it’s possible to sort strings by their length using a custom lambda function: lambda string: len(string).
Conclusion
Mastering the art of sorting strings in Python empowers you to organize and manipulate textual data with precision and efficiency. As your knowledge deepens, you’ll unlock the potential to analyze, process, and extract meaningful insights from vast collections of textual information.
Are you ready to embark on this alphabetical adventure with Python as your guide?