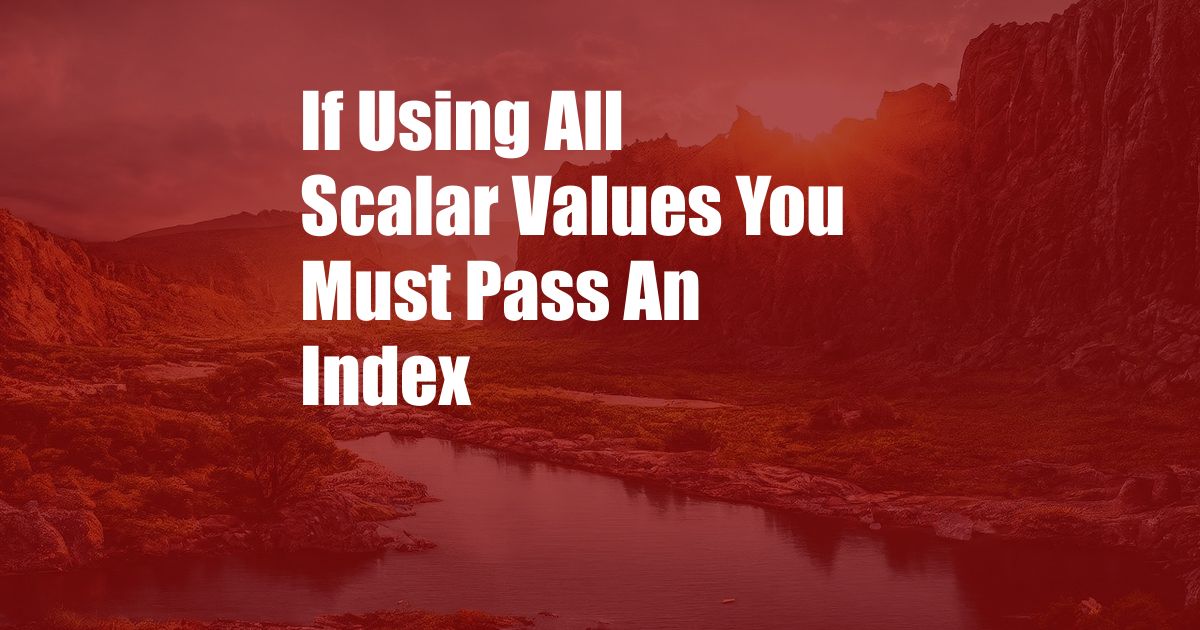
If Using All Scalar Values You Must Pass an Index
In the realm of programming, data structures are essential tools for organizing and managing information. Among the many data structures available, arrays are a fundamental and widely used structure that allows us to store a collection of elements of the same type.
An array is essentially a contiguous block of memory that holds elements in a sequential order. Each element in an array occupies a fixed amount of memory and is identified by its index, which represents its position within the array. Arrays provide efficient access to elements and offer various operations for manipulating the data they contain.
Understanding Indices in Arrays
Indices play a crucial role in working with arrays. Each element in an array is associated with a unique index that identifies its location. The index of the first element is typically 0, and the index of the last element is one less than the total number of elements in the array.
When accessing elements of an array, it is essential to specify the correct index. If the index is out of bounds (i.e., less than 0 or greater than or equal to the total number of elements), it can lead to undefined behavior or errors. Therefore, it is important to carefully consider the indices when working with arrays.
Why You Must Pass an Index When Using All Scalar Values
In certain programming languages, such as C and C++, passing an index is mandatory when using all scalar values in an array. This means that even if you want to access all elements of an array, you must still specify the index range.
This requirement is imposed because scalar values, such as integers and floating-point numbers, occupy only a single memory location. Without an index, the compiler cannot determine the location of the elements in the array. By passing an index, you explicitly specify the starting point of the array, allowing the compiler to calculate the addresses of subsequent elements.
Benefits of Enforcing Index Passing
Enforcing the passing of an index for all scalar values in arrays has several benefits:
- Enhanced Safety: It prevents out-of-bounds errors and ensures that you always access valid elements of the array.
- Improved Readability: By explicitly specifying the index range, the code becomes more readable and easier to understand.
- Compiler Optimization: In some cases, the compiler can optimize code that uses indices for accessing arrays, improving performance.
Tips for Working with Indices in Arrays
Here are some additional tips for effectively working with indices in arrays:
- Use Meaningful Indices: Instead of using arbitrary numbers, consider using descriptive names for indices to enhance code readability.
- Validate Indices: Always check if the indices are within the bounds of the array before accessing elements.
- Use Iterators Carefully: When using iterators to traverse arrays, ensure that they are properly initialized and incremented to avoid index errors.
Frequently Asked Questions (FAQ)
Conclusion
Passing an index when using all scalar values in arrays is an important practice in programming. By understanding the role of indices and the benefits of explicit index passing, you can effectively work with arrays and enhance the quality and safety of your code.
Remember, arrays are essential data structures that provide efficient and flexible ways to store and manage data. By mastering the use of indices, you can unlock the full potential of arrays and write robust and maintainable code.
Call to Action: If you have any further questions or require additional clarification on using indices in arrays, feel free to reach out for assistance.