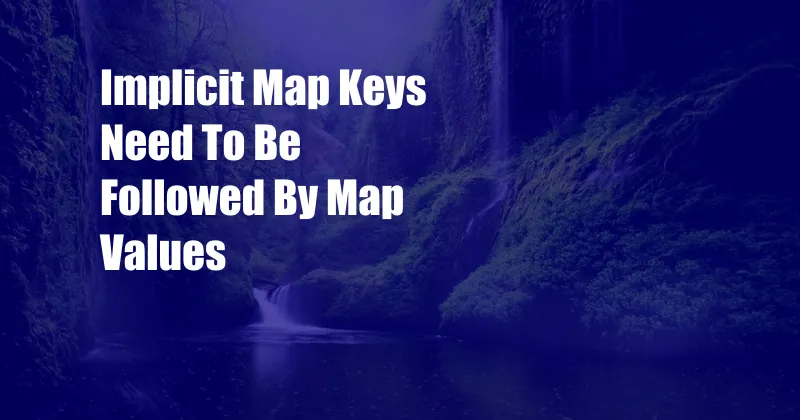
Implicit Map Keys Must Be Followed by Map Values
I recently ran into an issue where I was trying to use an implicit map key without providing a corresponding map value. This resulted in a confusing error message and took me some time to debug. In this blog post, I’ll explain what implicit map keys are, why they need to be followed by map values, and how to avoid this issue in your own code.
Implicit map keys are a shortcut syntax for creating a map. Instead of using the explicit syntax:
val myMap = Map("key1" -> "value1", "key2" -> "value2")
You can use the implicit syntax:
val myMap = Map("key1, "value1", "key2", "value2")
The implicit syntax is shorter and easier to read, but it can be confusing if you’re not aware of the rules for using it. One of the most important rules is that implicit map keys must be followed by map values.
Why Implicit Map Keys Need to Be Followed by Map Values
The reason why implicit map keys must be followed by map values is because the compiler needs to be able to distinguish between keys and values. If you don’t provide a map value, the compiler won’t know whether the key is a key or a value. This can lead to confusing error messages and unexpected behavior.
For example, the following code will result in a compiler error:
val myMap = Map("key1", "key2")
The error message will be:
error: missing argument for parameter value in
This error message is confusing because it doesn’t tell you what the missing argument is. The actual problem is that the compiler doesn’t know whether “key2” is a key or a value. To fix the error, you need to provide a map value:
val myMap = Map("key1" -> "value1", "key2" -> "value2")
How to Avoid This Issue
The best way to avoid this issue is to always use the explicit syntax for creating maps. This will make your code more readable and easier to debug. If you do need to use the implicit syntax, be sure to follow the rule that implicit map keys must be followed by map values.
Tips and Expert Advice
Here are some tips and expert advice for using implicit map keys:
- Only use implicit map keys if you’re sure that the compiler will be able to distinguish between keys and values.
- If you’re not sure whether the compiler will be able to distinguish between keys and values, use the explicit syntax instead.
- Be consistent in your use of implicit and explicit map keys. This will make your code more readable and easier to maintain.
FAQ
Here are some frequently asked questions about implicit map keys:
- What is the difference between an implicit map key and an explicit map key?
- Why do implicit map keys need to be followed by map values?
- How can I avoid this issue?
An implicit map key is a shortcut syntax for creating a map. Instead of using the explicit syntax:
val myMap = Map("key1" -> "value1", "key2" -> "value2")
You can use the implicit syntax:
val myMap = Map("key1, "value1", "key2", "value2")
The implicit syntax is shorter and easier to read, but it can be confusing if you’re not aware of the rules for using it.
Implicit map keys need to be followed by map values because the compiler needs to be able to distinguish between keys and values. If you don’t provide a map value, the compiler won’t know whether the key is a key or a value. This can lead to confusing error messages and unexpected behavior.
The best way to avoid this issue is to always use the explicit syntax for creating maps. This will make your code more readable and easier to debug. If you do need to use the implicit syntax, be sure to follow the rule that implicit map keys must be followed by map values.
Conclusion
Implicit map keys can be a useful shortcut, but it’s important to be aware of the rules for using them. Implicit map keys must be followed by map values, otherwise the compiler won’t be able to distinguish between keys and values. This can lead to confusing error messages and unexpected behavior.
Are you interested to know more?