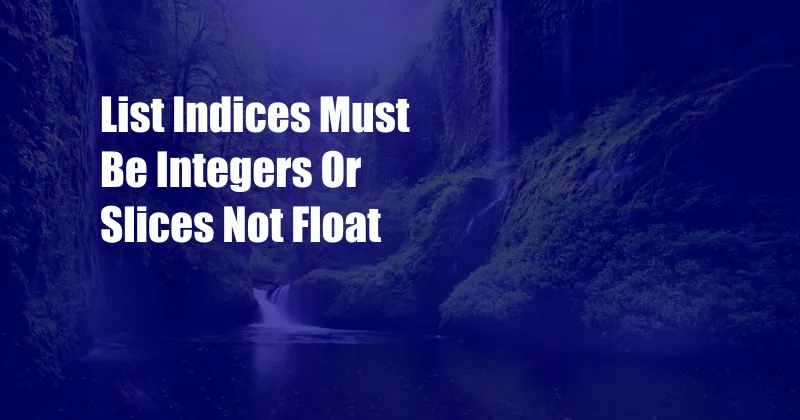
List Indices Must Be Integers or Slices, Not Float
When working with lists in Python, it’s important to remember that list indices must be integers or slices, not float. This is because lists are ordered collections of items, and each item in the list has an associated index. Indices are used to access specific items in the list, and they must be integers or slices that represent valid positions within the list.
Trying to use a float as a list index will result in a TypeError. For example, the following code will generate an error:
my_list = [1, 2, 3]
index = 1.5
item = my_list[index]
This error occurs because the index 1.5 is not a valid position within the list. Lists can only be indexed using integers or slices, so using a float as an index will result in an error.
When to Use Integers as List Indices
Integers are the most common type of list index. They are used to access specific items in the list, and they must be within the range of valid indices for the list. For example, the following code uses an integer index to access the second item in the list:
my_list = [1, 2, 3]
index = 1
item = my_list[index]
In this example, the index 1 is a valid position within the list, so the code will successfully retrieve the second item in the list (which is the number 2).
When to Use Slices as List Indices
Slices are used to access a range of items in a list. They are specified using the following syntax:
my_list[start:end]
The start and end values are integers that specify the starting and ending positions of the slice, respectively. The slice will include all items in the list from the start position up to, but not including, the end position. For example, the following code uses a slice to access the first three items in the list:
my_list = [1, 2, 3]
slice = my_list[0:3]
In this example, the slice [0:3] includes the items at indices 0, 1, and 2. The resulting slice would be a new list containing the numbers 1, 2, and 3.
Tips and Expert Advice
Here are some tips and expert advice for using list indices in Python:
- Use integers to access specific items in the list.
- Use slices to access a range of items in the list.
- Make sure that your indices are within the range of valid indices for the list.
- Avoid using float as list indices.
By following these tips, you can ensure that you are using list indices correctly and efficiently in your Python code.
Explanation of Tips and Expert Advice
The tips and expert advice above are designed to help you use list indices correctly and efficiently in your Python code. Here is a brief explanation of each tip:
- **Use integers to access specific items in the list.** Integers are the most common type of list index because they allow you to access specific items in the list. For example, the following code uses an integer index to access the second item in the list:
my_list = [1, 2, 3] index = 1 item = my_list[index]
In this example, the index 1 is a valid position within the list, so the code will successfully retrieve the second item in the list (which is the number 2).
- **Use slices to access a range of items in the list.** Slices are used to access a range of items in a list. They are specified using the following syntax:
my_list[start:end]
The start and end values are integers that specify the starting and ending positions of the slice, respectively. The slice will include all items in the list from the start position up to, but not including, the end position. For example, the following code uses a slice to access the first three items in the list:
my_list = [1, 2, 3] slice = my_list[0:3]
In this example, the slice [0:3] includes the items at indices 0, 1, and 2. The resulting slice would be a new list containing the numbers 1, 2, and 3.
- **Make sure that your indices are within the range of valid indices for the list.** List indices must be within the range of valid indices for the list. The valid indices for a list are the integers from 0 to len(list) – 1. Trying to use an index that is outside of this range will result in an error. For example, the following code will generate an error because the index 3 is outside of the range of valid indices for the list:
my_list = [1, 2, 3] index = 3 item = my_list[index]
This error occurs because the index 3 is not a valid position within the list. The list only contains three items, so the valid indices are 0, 1, and 2.
- **Avoid using float as list indices.** Float should not be used as list indices because they can lead to unexpected results. For example, the following code will generate an error because the index 1.5 is not a valid position within the list:
my_list = [1, 2, 3] index = 1.5 item = my_list[index]
This error occurs because the index 1.5 is not an integer. List indices must be integers or slices, so using a float as an index will result in an error.
FAQ
Q: Why can’t I use float as list indices?
A: Float cannot be used as list indices because they can lead to unexpected results. List indices must be integers or slices, so using a float as an index will result in an error.
Q: What is the difference between an integer index and a slice?
A: An integer index accesses a specific item in the list, while a slice accesses a range of items in the list. Integer indices are specified using a single integer, while slices are specified using the following syntax: my_list[start:end].
Q: How do I make sure that my indices are within the range of valid indices for the list?
A: You can make sure that your indices are within the range of valid indices for the list by using the len() function. The len() function returns the number of items in the list, so you can use it to determine the highest valid index for the list.
Conclusion
List indices must be integers or slices, not float. This is because lists are ordered collections of items, and each item in the list has an associated index. Indices are used to access specific items in the list, and they must be integers or slices that represent valid positions within the list. By following the tips and advice in this article, you can ensure that you are using list indices correctly and efficiently in your Python code.
Are you interested in learning more about list indices in Python? If so, I encourage you to check out the following resources: