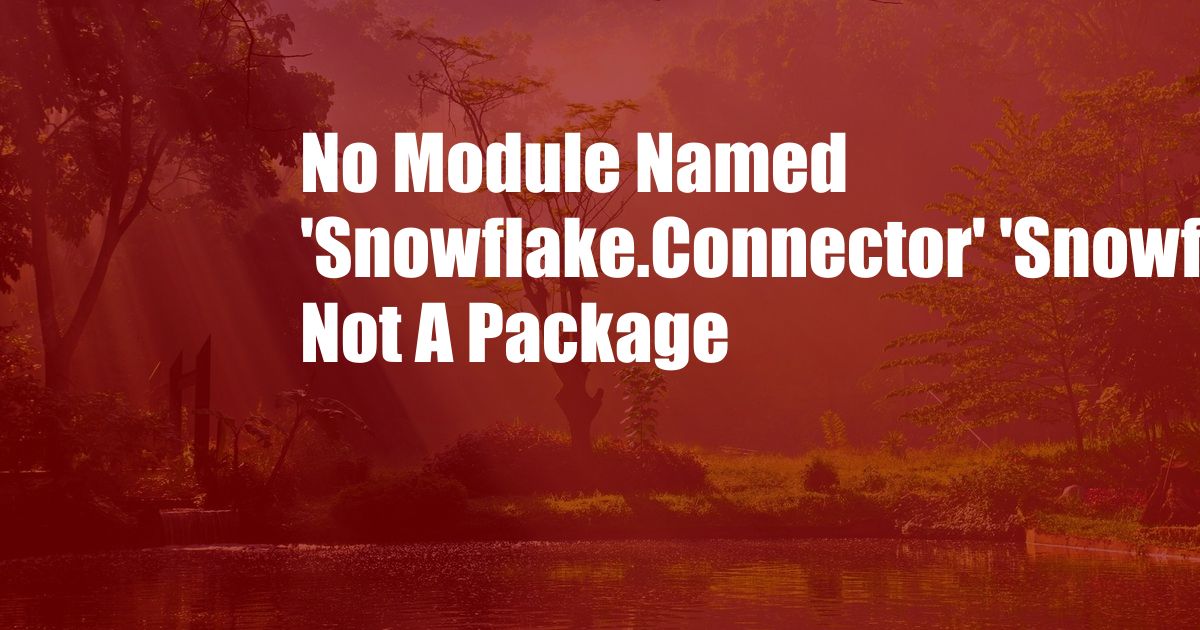
no module named ‘snowflake.connector’ ‘snowflake’ is not a package
The error message “no module named ‘snowflake.connector’ ‘snowflake’ is not a package” occurs when you try to import the snowflake. connector module in Python, but the module is not installed or not installed correctly.
To resolve this error, you can install the snowflake-connector-python package using pip:
pip install snowflake-connector-python
Once the package is installed, you should be able to import the snowflake. connector module without encountering the error.
Installing the snowflake-connector-python Package
Once the snowflake-connector-python package is installed, you should be able to import the snowflake.connector module without encountering the error.
Here’s an example of how to import the snowflake.connector module:
import snowflake.connector
Once the module is imported, you can use it to connect to a Snowflake database and execute SQL queries.
Overview of the snowflake.connector Module
The snowflake.connector module is a Python package that allows you to connect to a Snowflake database and execute SQL queries.
The module provides a set of classes and methods that make it easy to connect to a Snowflake database, execute SQL queries, and retrieve the results.
Key Features of the snowflake.connector Module
The snowflake.connector module offers several key features, including:
- Support for connecting to Snowflake databases using a variety of authentication methods, including password, OAuth, and Azure Active Directory.
- The ability to execute SQL queries and retrieve the results.
- Support for binding parameters to SQL queries.
- The ability to create and manage cursors.
- Support for handling transactions.
ul>
The snowflake.connector module is a powerful tool that can be used to interact with Snowflake databases from Python applications.
Example of Using the snowflake.connector Module
Here’s an example of how to use the snowflake.connector module to connect to a Snowflake database and execute a SQL query:
import snowflake.connector
# Create a connection to the Snowflake database
cnx = snowflake.connector.connect(
user='my_user',
password='my_password',
account='my_account',
)
# Create a cursor
cur = cnx.cursor()
# Execute a SQL query
cur.execute("SELECT * FROM my_table")
# Fetch the results
results = cur.fetchall()
# Print the results
for row in results:
print(row)
# Close the cursor and connection
cur.close()
cnx.close()
This code will connect to the Snowflake database specified by the user, password, and account parameters. It will then execute the SQL query “SELECT * FROM my_table” and print the results.
Conclusion
The snowflake.connector module is a powerful tool that allows you to connect to a Snowflake database and execute SQL queries from Python applications.
The module is easy to use and provides a comprehensive set of features. It is a valuable tool for anyone who needs to interact with Snowflake databases from Python.
Are you interested in learning more about the snowflake.connector module? Let us know in the comments below!
FAQ
Q: How do I install the snowflake.connector module?
A: You can install the snowflake.connector module using pip:
pip install snowflake-connector-python
Q: How do I connect to a Snowflake database using the snowflake.connector module?
A: To connect to a Snowflake database using the snowflake.connector module, you can use the following code:
import snowflake.connector
# Create a connection to the Snowflake database
cnx = snowflake.connector.connect(
user='my_user',
password='my_password',
account='my_account',
)
Q: How do I execute a SQL query using the snowflake.connector module?
A: To execute a SQL query using the snowflake.connector module, you can use the following code:
# Create a cursor
cur = cnx.cursor()
# Execute a SQL query
cur.execute("SELECT * FROM my_table")