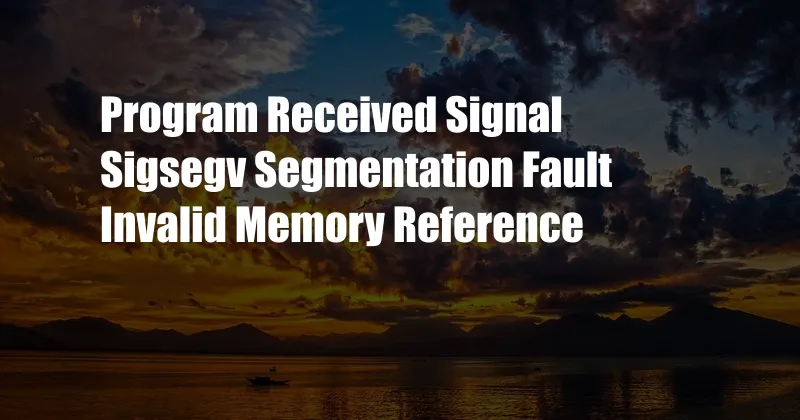
Program Received Signal SIGSEGV: Segmentation Fault (Invalid Memory Reference)
I was working on a C++ project when suddenly my program crashed with a segmentation fault error. The error message was: “Program received signal SIGSEGV: Segmentation fault (invalid memory reference).” I had never encountered this error before, so I was a bit confused about what it meant and how to fix it.
Understanding Segmentation Faults
A segmentation fault occurs when a program tries to access memory that it is not allowed to access. This can happen for a number of reasons, such as:
- Out-of-bounds array access: Trying to access an element of an array that is beyond the bounds of the array.
- Dereferencing a null pointer: Trying to access the memory location pointed to by a null pointer.
- Double freeing: Freeing the same memory location twice.
- Buffer overflow: Writing more data to a buffer than it can hold, causing data to overflow into adjacent memory locations.
Debugging Segmentation Faults
Debugging segmentation faults can be a bit tricky, as the error message doesn’t always provide a lot of information about the cause of the fault. Here are a few tips for debugging segmentation faults:
- Use a debugger: A debugger can help you step through your code and inspect the memory state at each step. This can help you identify the specific line of code that is causing the fault.
- Check your array bounds: Make sure that you are not accessing elements of arrays that are beyond the bounds of the array.
- Check your pointers: Make sure that you are not dereferencing null pointers.
- Check for buffer overflows: Make sure that you are not writing more data to a buffer than it can hold.
- Use memory debugging tools: There are a number of memory debugging tools that can help you detect memory leaks and other memory-related errors.
Preventing Segmentation Faults
The best way to prevent segmentation faults is to write clean and well-tested code. Here are a few tips for preventing segmentation faults:
- Use bounds checking: Always check the bounds of arrays before accessing them.
- Use null checks: Always check pointers for null before dereferencing them.
- Use memory management tools: Use memory management tools to help you keep track of allocated memory and prevent memory leaks.
- Test your code thoroughly: Test your code thoroughly to make sure that it is working as expected.
- Use a static analyzer: A static analyzer can help you find potential memory errors in your code.
Troubleshooting Common Segmentation Fault Situations
Here are a few common segmentation fault situations and how to troubleshoot them:
- Out-of-bounds array access: This can be caused by a number of things, such as a typo in the array index, or a loop that iterates beyond the bounds of the array. To fix this, check your array bounds and make sure that you are accessing the array correctly.
- Dereferencing a null pointer: This can be caused by a number of things, such as a null pointer being passed to a function, or a pointer being set to null after it has been dereferenced. To fix this, check your pointers for null before dereferencing them.
- Double freeing: This can be caused by freeing the same memory location twice, either directly or indirectly. To fix this, make sure that you are only freeing each memory location once.
- Buffer overflow: This can be caused by writing more data to a buffer than it can hold. To fix this, make sure that you are not writing more data to a buffer than it can hold.
Conclusion
Segmentation faults are a common error in C++ programming. By understanding the causes of segmentation faults and how to debug them, you can prevent them from occurring in your code. Remember to use bounds checking, null checks, memory management tools, and thorough testing to keep your code clean and error-free.
FAQ
Q: What is a segmentation fault?
A: A segmentation fault is an error that occurs when a program tries to access memory that it is not allowed to access.
Q: What causes a segmentation fault?
A: Segmentation faults can be caused by a number of things, such as out-of-bounds array access, dereferencing a null pointer, double freeing, and buffer overflows.
Q: How can I debug a segmentation fault?
A: You can debug a segmentation fault by using a debugger, checking your array bounds, checking your pointers, checking for buffer overflows, and using memory debugging tools.
Q: How can I prevent segmentation faults?
A: You can prevent segmentation faults by using bounds checking, null checks, memory management tools, and thorough testing.
Q: Are you interested in reading more about program received signal SIGSEGV: Segmentation fault (invalid memory reference)?
A: If you are interested in reading more about program received signal SIGSEGV: Segmentation fault (invalid memory reference), please let me know, I will be happy to provide you with more information.