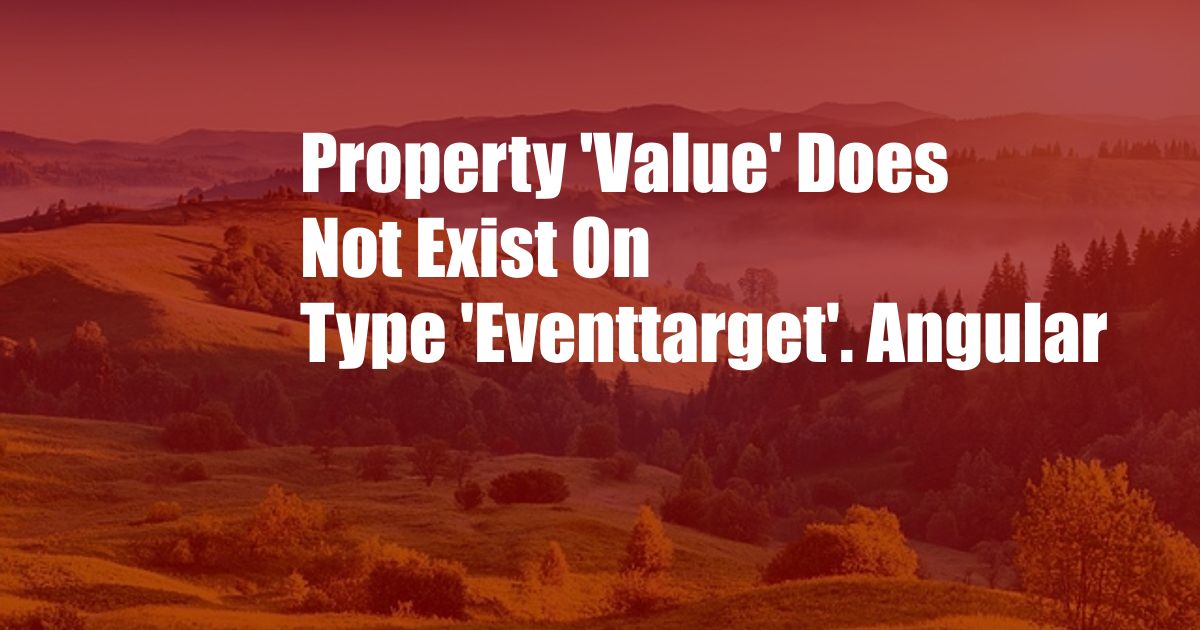
Property ‘value’ does not exist on type ‘EventTarget’
In Angular, when accessing form controls within event handlers, you may encounter the error “Property ‘value’ does not exist on type ‘EventTarget.'” This error occurs because the event object passed to event handlers in Angular does not have a direct reference to the form control’s value property. To resolve this issue, we need to explicitly extract the value from the event object.
This issue commonly arises when working with event-emitting elements like input fields, where you intend to capture the value entered by the user. Without proper handling, attempting to access the ‘value’ property directly from within event handlers can lead to the error mentioned above.
Accessing Form Control Value
To obtain the value of a form control within an event handler, you can employ one of two approaches. The first method involves using the ‘target’ property of the event object to access the underlying DOM element and retrieve its value. This approach is commonly employed when dealing with native HTML elements:
<input type="text" (input)="onInput($event)">
onInput(event: Event) const value = (event.target as HTMLInputElement).value; // Use the value as needed
Alternatively, in scenarios where the event originates from Angular-specific form controls like ‘FormControl’ or ‘FormGroup,’ we can leverage the ‘value’ property of the form control itself. This approach simplifies the process:
<input formControlName="username" (input)="onInput($event)">
onInput(event: Event) const formControl = event.target as FormControl; const value = formControl.value; // Use the value as needed
By utilizing either of these approaches, you can effectively retrieve the form control’s value within event handlers and proceed with the necessary operations.
Understanding the ‘EventTarget’ Type
In Angular applications, event handlers receive an object of type ‘EventTarget’ as their first argument. The ‘EventTarget’ type represents the element that triggered the event. It provides access to event-related information and properties, but not directly to the form control’s ‘value’ property.
To access the form control’s ‘value’ property, we need to cast the ‘EventTarget’ object to a more specific type, such as ‘HTMLInputElement’ for native HTML elements or ‘FormControl’ for Angular form controls. This allows us to access the properties and methods specific to the target element and retrieve the ‘value’ property as needed.
Tips and Expert Advice
Here are some additional tips and expert advice to help you work with form control values in Angular event handlers:
- Always ensure that you are casting the ‘EventTarget’ object to the correct type. This ensures proper access to the ‘value’ property and prevents errors.
- Consider using template-driven forms whenever possible. Template-driven forms simplify accessing form control values by automatically binding them to the component’s properties.
- For complex scenarios, you can use custom event emitters to pass form control values from child components to parent components.
- Keep your event handlers concise and focused. Avoid performing heavy operations or complex logic within event handlers.
Frequently Asked Questions
Q: Why do I get the “Property ‘value’ does not exist on type ‘EventTarget'” error?
A: This error occurs because the event object passed to event handlers does not have a direct reference to the form control’s ‘value’ property. You need to explicitly cast the ‘EventTarget’ object to the correct type to access the ‘value’ property.
Q: What is the best approach to retrieve form control values in Angular event handlers?
A: You can either use the ‘target’ property of the event object to access the underlying DOM element and retrieve its value, or you can leverage the ‘value’ property of the form control itself if the event originates from an Angular-specific form control.
Q: What is the ‘EventTarget’ type?
A: The ‘EventTarget’ type represents the element that triggered an event in Angular applications. It provides access to event-related information and properties, but not directly to the form control’s ‘value’ property.
Q: How do I cast the ‘EventTarget’ object to the correct type?
A: You can cast the ‘EventTarget’ object to a more specific type, such as ‘HTMLInputElement’ for native HTML elements or ‘FormControl’ for Angular form controls. This allows you to access the properties and methods specific to the target element and retrieve the ‘value’ property as needed.
Conclusion
Understanding how to access form control values in Angular event handlers is crucial for building dynamic and interactive user interfaces. By following the guidance provided in this article, you can effectively retrieve form control values and enhance the functionality of your Angular applications. If you have any further questions or require additional insights, feel free to explore online resources, forums, and documentation to deepen your understanding of this topic.