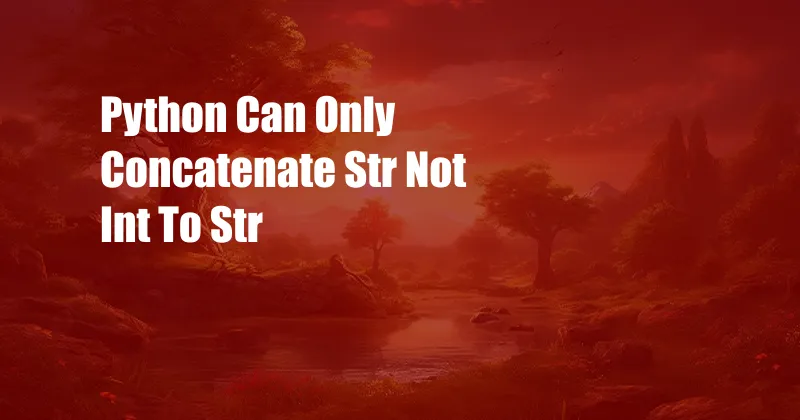
Python: Unveiling the Secrets of String Concatenation
During my coding journey, I encountered a puzzling error while attempting to combine strings and integers. Python’s unwavering stance on concatenating only strings left me baffled. Intrigued by this limitation, I delved into the depths of Python’s design principles, eager to unravel the mysteries behind this behavior.
To fully grasp the intricacies of string concatenation, let’s explore the concept itself. In the realm of programming, concatenation refers to the process of joining two or more strings together, often used to create larger, more comprehensive texts. In Python, the “+” operator serves as the magical wand for string concatenation.
The Riddle of Int-String Concatenation
The enigma surrounding Python’s refusal to concatenate integers and strings stems from its strict adherence to data type consistency. Python, being a strongly typed language, demands that operands in an operation share the same data type. When confronted with an attempt to concatenate an integer (e.g., 123) and a string (e.g., “Hello”), Python raises an error, signaling its disapproval of such a union.
To illustrate this concept, let’s embark on a Pythonic adventure with the following code snippet:
>>> "Hello" + 123
TypeError: can only concatenate str (not "int") to str
As you can witness, Python remains resolute in its stance, refusing to compromise its data type integrity.
Overcoming the Concatenation Obstacle
Fear not, dear Pythonistas! There are ways to bypass this obstacle and achieve the desired string concatenation. The key lies in converting the integer into a string before attempting the union. Python provides a handy function, aptly named `str()`, which effortlessly transforms integers into their string counterparts. By embracing this conversion technique, we can gracefully concatenate strings and integers, harmoniously blending them into a single, cohesive text.
To exemplify the power of `str()`, let’s revisit our earlier code snippet:
>>> "Hello" + str(123)
'Hello123'
Behold! With the integer successfully converted to a string, Python happily complies, producing the concatenated result, “Hello123”.
Expert Advice: Embracing Best Practices
As we navigate the world of Python, it’s prudent to adopt best practices that enhance code readability and maintainability. When dealing with string concatenation, consider the following expert advice:
- Utilize the `join()` method: For concatenating multiple strings, the `join()` method offers a concise and efficient solution. Simply pass the desired strings as a list to `join()`, and it will seamlessly merge them together.
- Leverage string interpolation: Python’s string interpolation feature empowers you to embed expressions directly within strings. This technique allows for dynamic string creation, making your code more concise and expressive.
- Apply the `format()` method: The `format()` method provides a powerful mechanism for formatting strings. By leveraging placeholders and keyword arguments, you can dynamically generate complex strings with ease.
By adhering to these best practices, you can elevate your Python coding skills, unlocking new levels of efficiency and clarity.
FAQ: Demystifying Concatenation
To further illuminate the topic, let’s delve into some frequently asked questions:
- Q: Can I concatenate a list of strings?
A: Absolutely! Python’s `join()` method is your trusted ally for this task. Simply pass the list of strings to `join()`, and it will effortlessly combine them into a single string. - Q: How do I concatenate a string multiple times?
A: Python provides the `*` operator for string repetition. Simply multiply the string by the desired number of repetitions. For instance, “Hello” * 3 yields “HelloHelloHello”. - Q: Is there a limit to string concatenation?
A: Python imposes no inherent limit on string concatenation. However, excessively large strings may encounter memory constraints, so exercise caution when dealing with massive string operations.
Conclusion
In the realm of Python, string concatenation stands as a fundamental operation, unlocking the power to seamlessly combine strings and create larger, more meaningful texts. While Python’s strict data type adherence may initially seem like a hindrance, embracing the `str()` function provides a simple and effective workaround. By adhering to best practices and delving into expert advice, you can master the art of string concatenation, elevating your Pythonic prowess to new heights.
Now, dear readers, I invite you to embark on a journey of discovery. Delve deeper into the world of string concatenation, explore its nuances, and uncover its potential to empower your Pythonic creations. Share your insights and experiences in the comments below, fostering a vibrant community of knowledge seekers.