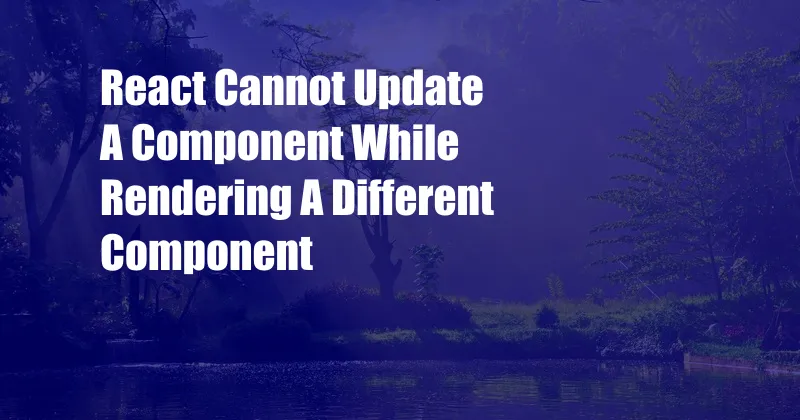
React: Handling Updates While Rendering
In the dynamic world of React applications, it’s essential to maintain a smooth and efficient user experience. One crucial aspect of this is understanding how component updates are handled during the rendering process. In this article, we’ll delve into the intricacies of React’s update mechanism and provide tips to avoid common pitfalls.
Before we dive into the details, let’s paint a vivid picture through a personal anecdote. Imagine you’re working on a real-time chat application that involves updating user profiles and message history simultaneously. You render the user profiles on the left panel and the message history on the right. As a user starts typing, the input field triggers a state update in the message history component. However, you notice that the user profile component also updates unexpectedly, leading to a jarring and confusing interface.
The Concurrency of Updates
To understand why this happens, we need to delve into the concurrency of React’s update mechanism. React embraces a non-blocking approach, meaning that state updates are scheduled asynchronously and do not immediately trigger a re-render. Instead, they are encapsulated in a queue, and a single re-render is performed once the queue is flushed. This approach enhances performance by preventing unnecessary re-renders and ensuring that updates are efficiently processed.
Impact on Concurrent Updates
However, this concurrency can lead to unexpected behavior when multiple components are updated simultaneously. When a component is in the middle of rendering, it creates a temporary snapshot of its state. If another component triggers an update during this rendering phase, the updated state is not immediately reflected in the snapshot. This can result in the component being rendered with an outdated state, leading to the aforementioned issue in our chat application example.
Avoiding Concurrent Update Pitfalls
To avoid these pitfalls and ensure predictable updates, it’s important to understand the following principles:
- Controlled Updates: Updates should be controlled through a centralized mechanism, such as a Redux store or React’s useState hook, to prevent multiple components from updating the same state simultaneously.
- State Management: Use immutable state management techniques to avoid accidental mutations. Immutability ensures that updates are predictable and do not lead to unintended side effects.
- Debouncing and Throttling: Implement debouncing or throttling techniques to limit the frequency of updates, especially when triggered by user inputs. This prevents unnecessary re-renders and enhances performance.
Tips for Smooth Updates
In addition to the principles mentioned above, here are some expert tips to ensure smooth updates in React applications:
- Use the latest version of React and follow recommended best practices.
- Create custom hooks to manage state and updates, promoting code reusability and maintainability.
- Leverage the React Profiler tool to identify performance bottlenecks and optimize updates accordingly.
FAQ
Q: Can I update a component while rendering another component?
A: No, it is not recommended to update a component while rendering another component. This can lead to unexpected behavior and inconsistent state management.
Q: How can I ensure that my updates are always predictable?
A: By adhering to controlled updates, state management principles, and using debouncing or throttling techniques, you can enhance the predictability of your updates.
Q: What tools can I use to identify and resolve update-related issues?
A: Utilize the React Profiler tool to pinpoint performance bottlenecks and identify areas for optimization.
Conclusion
Mastering the intricacies of React’s update mechanism is crucial for building efficient and responsive applications. By following the principles and tips outlined in this article, you can avoid common pitfalls and ensure that your updates are handled predictably and efficiently. Remember, a well-managed update process not only enhances the user experience but also lays the foundation for scalable and maintainable React applications.
Are you ready to dive deeper into the world of React updates and take your application development skills to the next level?