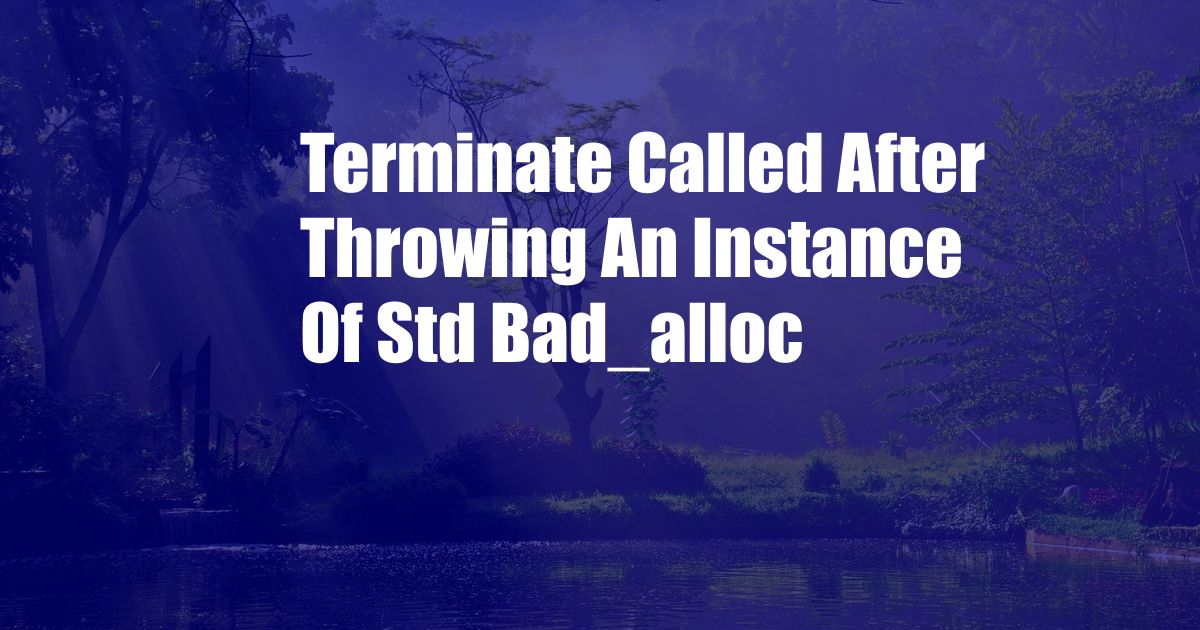
Terminate Called After Throwing an Instance of Std Bad Alloc: An In-Depth Exploration
In the depths of software development, I once encountered a cryptic error message that left me perplexed: “terminate called after throwing an instance of ‘std::bad_alloc’.” This enigmatic message hinted at an underlying issue with memory allocation, threatening to derail my programming progress. Determined to unravel its meaning, I embarked on a journey into the realm of memory management, encountering valuable lessons along the way.
Before diving into the technical details, let’s pause to understand the significance of memory allocation in programming. Memory allocation is the process by which a program requests and acquires memory from the operating system to store data and instructions. When a program tries to allocate memory but fails due to insufficient resources, it throws an instance of “std::bad_alloc,” resulting in the infamous “terminate called after throwing an instance of ‘std::bad_alloc'” error message.
Understanding Memory Allocation
To comprehend the “terminate called after throwing an instance of ‘std::bad_alloc'” error message, it’s crucial to grasp the concept of memory allocation. Memory allocation is the act of requesting and acquiring memory from the operating system to store data and instructions for a program. This process ensures that the program has the necessary resources to execute its tasks.
Memory allocation is typically handled by the operating system’s memory manager, which maintains a pool of available memory. When a program requests memory, the memory manager allocates a block of memory from the pool and assigns it to the program. This allocated memory can then be used by the program to store data and instructions.
The Role of “std::bad_alloc”
“std::bad_alloc” is a standard C++ exception that is thrown when a memory allocation request fails. This can occur due to several reasons, including:
- Insufficient memory: The operating system has run out of available memory to allocate to the program.
- Fragmented memory: The available memory is fragmented into small blocks, making it difficult to allocate a large contiguous block of memory.
- Memory leaks: The program has allocated memory that is no longer in use but has not been released, leading to a gradual depletion of available memory.
Resolving Memory Allocation Issues
To resolve memory allocation issues, several strategies can be employed:
- Handle memory allocation errors gracefully: Implement robust error handling mechanisms to catch and handle memory allocation errors gracefully. This involves checking for “std::bad_alloc” exceptions and taking appropriate actions, such as retrying the allocation or notifying the user.
- Optimize memory usage: Review the program’s memory usage patterns and identify areas where memory can be optimized. This includes reducing memory fragmentation, avoiding memory leaks, and using appropriate data structures.
- Monitor memory usage: Implement mechanisms to monitor the program’s memory usage and detect potential memory problems early on. This can help identify memory leaks and other issues before they cause significant problems.
Conclusion
The “terminate called after throwing an instance of ‘std::bad_alloc'” error message is a common issue encountered by programmers. By understanding the underlying causes of this error and implementing robust memory management strategies, developers can avoid or resolve these issues effectively. By optimizing memory usage, handling memory allocation errors gracefully, and monitoring memory usage, developers can ensure that their programs run reliably and efficiently.
Are you interested in learning more about memory management and resolving memory allocation errors? If so, I encourage you to explore the vast array of resources available online. Forums, tutorials, and documentation can provide valuable insights and help you become a proficient software developer capable of handling memory-related challenges with confidence.
Frequently Asked Questions (FAQs)
Q: Why does my program throw a “std::bad_alloc” exception?
A: A “std::bad_alloc” exception is thrown when the program attempts to allocate memory but fails due to insufficient memory, fragmented memory, or memory leaks.
Q: How can I handle “std::bad_alloc” exceptions gracefully?
A: Implement error handling mechanisms to catch and handle “std::bad_alloc” exceptions. Retry the allocation or notify the user, providing a meaningful error message.
Q: How can I optimize my program’s memory usage?
A: Review memory usage patterns, reduce memory fragmentation, avoid memory leaks, and use appropriate data structures to optimize memory usage.
Q: What is the best way to monitor memory usage in my program?
A: Implement mechanisms to monitor memory usage, such as using memory profilers or debugging tools, to detect potential memory problems early on.
Q: Where can I find more resources on memory management?
A: Explore online forums, tutorials, and documentation for in-depth discussions and guidance on memory management and resolving memory allocation errors.