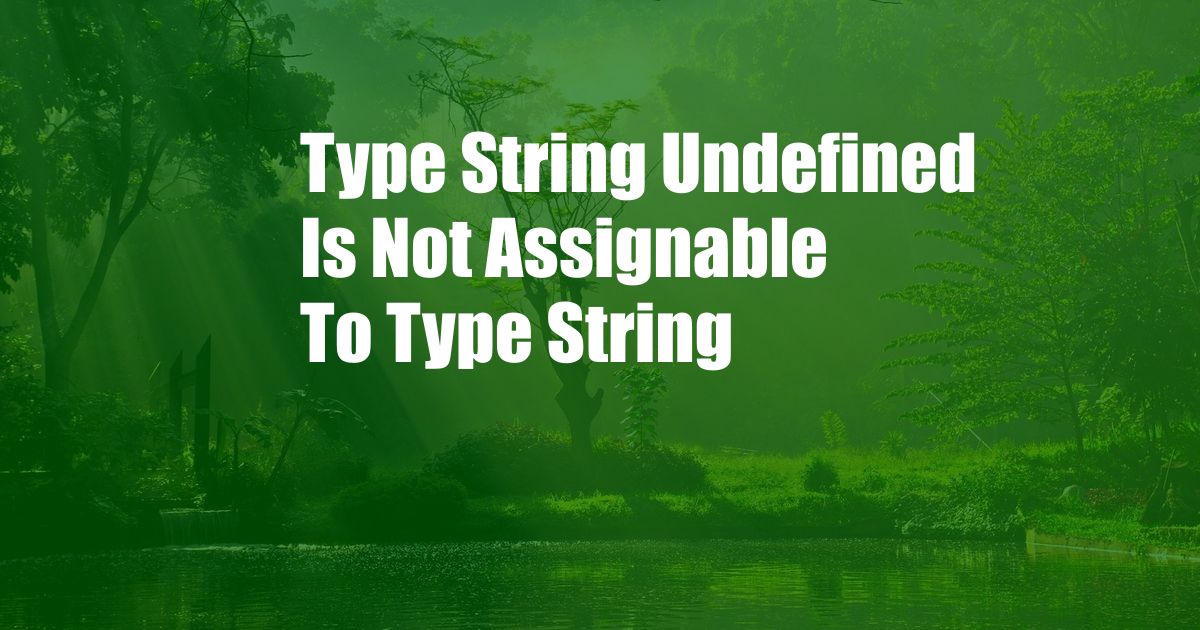
type string undefined is not assignable to type string
As a blogger, I often encounter various technical challenges while coding. One such challenge that I recently faced was the “type string undefined is not assignable to type string” error message. Initially, it puzzled me, but after some research and debugging, I was able to understand the cause and find a solution. In this article, I aim to share my experience and provide a comprehensive overview of this error, its causes, and effective troubleshooting methods.
Before delving into the technical details, let’s first clarify the context of this error message. In JavaScript, TypeScript, and other programming languages, we often work with different data types such as strings, numbers, booleans, and arrays. Each data type has specific characteristics and can be assigned to variables accordingly. However, when we attempt to assign a value of one data type to a variable of another data type, we may encounter type errors.
Understanding Type Compatibility
To understand the “type string undefined is not assignable to type string” error, we need to delve into the concept of type compatibility. In TypeScript, type compatibility ensures that the data type of a value matches the expected data type of a variable or function parameter. This helps prevent errors and ensures the integrity of the codebase.
In our specific case, the error message indicates that we are trying to assign a value of type undefined to a variable of type string. This is not allowed because undefined is a special value that represents the absence of a value, while a string is a sequence of characters. Assigning undefined to a string variable would result in a type mismatch.
Causes of the Error
There are several common scenarios that can lead to the “type string undefined is not assignable to type string” error:
- Uninitialized Variables: When a variable is declared but not assigned a value, it is initialized with the undefined value. Attempting to use such a variable in a string context will trigger the error.
- Null Values: Null is a special value that represents the intentional absence of a value. Assigning null to a string variable will also result in a type error.
- Incorrect Type Casting: TypeScript allows us to cast values from one type to another using the
as
keyword. However, if we attempt to cast an undefined or null value to a string, the compiler will throw a type error.
- Third-Party Libraries: Sometimes, we may encounter this error when using third-party libraries or frameworks. These libraries may have their own type definitions that do not align with our project’s type definitions, leading to type errors.
Resolving the Error
To resolve the “type string undefined is not assignable to type string” error, we need to identify the cause and apply appropriate corrective measures:
- Initialize Variables: Always initialize variables with appropriate values to avoid undefined values.
- Handle Null Values: Check for null values before using them in string contexts. You can use the
if (value !== null)
condition to handle null values.
- Use Type Guards: Type guards allow you to check the type of a value at runtime. You can use the
typeof
operator or the
instanceof
operator to perform type checks.
- Update Type Definitions: If you are using third-party libraries, ensure that you have the latest type definitions installed. You can use the
npm install --save-dev @types/library-name
command to install type definitions.
Additional Tips
In addition to the troubleshooting steps mentioned above, here are some additional tips to help you avoid the “type string undefined is not assignable to type string” error:
- Use Strict Mode: Enabling strict mode in TypeScript can help you catch type errors early on during development.
- Use Type Annotations: Adding type annotations to your variables and function parameters can help the TypeScript compiler identify and report type errors more accurately.
- Read Error Messages Carefully: The error message often provides valuable information about the cause of the error. Read the error message carefully and try to understand the context in which the error occurred.
- Seek Help from the Community: If you are unable to resolve the error on your own, don’t hesitate to seek help from online forums, communities, or the TypeScript documentation.
Frequently Asked Questions (FAQs)
- Q: Why do I get the “type string undefined is not assignable to type string” error?
A: This error occurs when you try to assign a value of type undefined to a variable of type string. - Q: How can I fix this error?
A: To fix this error, initialize variables with appropriate values, handle null values, use type guards, update type definitions, and enable strict mode. - Q: Is it possible to assign undefined to a string variable?
A: No, you cannot assign undefined to a string variable because it is a type mismatch. - Q: What is the difference between undefined and null?
A: Undefined represents the absence of a value due to uninitialization, while null represents the intentional absence of a value.
Conclusion
The “type string undefined is not assignable to type string” error is a common TypeScript error that can be easily resolved by understanding the concept of type compatibility and implementing appropriate troubleshooting methods. By following the tips and advice outlined in this article, you can effectively avoid this error and improve the quality of your TypeScript code.
If you have any further questions or would like to share your experiences with this error, please feel free to leave a comment below. Together, we can continue to learn and grow as developers.