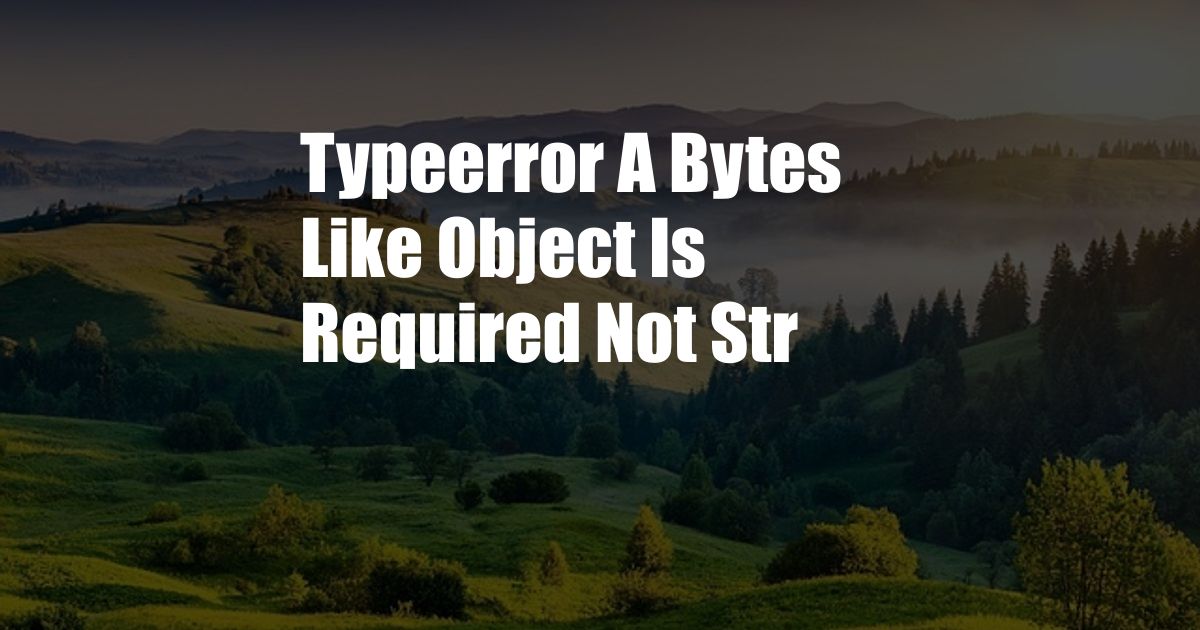
TypeError: a bytes-like object is required, not ‘str’
Have you encountered the “TypeError: a bytes-like object is required, not ‘str'” error while working with Python? This error can be frustrating to debug, especially for beginners. In this article, we’ll delve into the causes of this error and provide practical solutions to help you resolve it.
Before diving into the technical details, let’s start with a simple analogy to understand the underlying issue. Imagine you have a function that expects a certain type of input, such as a number. If you accidentally provide a string instead, the function will fail with an error message similar to “TypeError: a number is required, not ‘str'”. The “TypeError: a bytes-like object is required, not ‘str'” error follows the same principle.
What Causes the “TypeError: a bytes-like object is required, not ‘str'” Error?
In Python, there are two distinct data types: strings and bytes. Strings are sequences of Unicode characters represented by the ‘str’ type, while bytes are sequences of 8-bit values represented by the ‘bytes’ type. These two types are not interchangeable, and functions that expect bytes will fail if provided with strings.
The most common cause of the “TypeError: a bytes-like object is required, not ‘str'” error is attempting to use a string where a bytes-like object is required. This typically occurs when working with functions that deal with binary data, such as file I/O, encryption, or network communication. For example, the following code snippet will raise the error:
with open('myfile.txt', 'rb') as f:
data = f.read()
print(data)
In this example, the ‘open’ function expects a bytes-like object for the file path argument. Since ‘myfile.txt’ is a string, the code will fail with the “TypeError: a bytes-like object is required, not ‘str'” error.
How to Resolve the “TypeError: a bytes-like object is required, not ‘str'” Error
Resolving the “TypeError: a bytes-like object is required, not ‘str'” error requires converting the string to a bytes-like object before using it with the function. There are several ways to do this, depending on your specific requirements:
- Use the ‘encode()’ method: The ‘str’ type provides an ‘encode()’ method that converts a string to a bytes-like object. You can specify the encoding format as an argument, such as ‘utf-8’ or ‘ascii’.
- Use the ‘bytes()’ function: The ‘bytes()’ function can be used to create a bytes-like object from a string. You can pass the string as an argument, along with the optional encoding format.
- Use raw strings: Raw strings are prefixed with ‘rb’ or ‘rU’ to indicate that they should be treated as bytes-like objects. This can be useful when working with file paths or other string literals that represent binary data.
Tips and Expert Advice
Here are some additional tips and expert advice to help you avoid and resolve the “TypeError: a bytes-like object is required, not ‘str'” error:
- Understand the difference between strings and bytes: Familiarize yourself with the different data types and their intended uses.
- Check the documentation carefully: Before using any function, thoroughly review its documentation to determine the expected input types.
- Use type annotations: Python 3.6 and later support type annotations, which can help identify potential type errors at development time.
- Use a debugger: If you encounter the error, consider using a debugger to step through the code and identify the exact point where the conversion is needed.
FAQ
- Q: Why do I get the “TypeError: a bytes-like object is required, not ‘str'” error when using a string?
A: This error occurs because the function you are using expects a bytes-like object, such as a ‘bytes’ object or a raw string, but you are providing a regular string.
- Q: How can I convert a string to a bytes-like object?
A: You can use the ‘encode()’ method on the ‘str’ type, the ‘bytes()’ function, or use raw strings (prefixed with ‘rb’ or ‘rU’).
- Q: What is the difference between a bytes-like object and a string?
A: Bytes-like objects represent sequences of 8-bit values, while strings represent sequences of Unicode characters. Bytes-like objects are used for binary data, while strings are used for text.
Conclusion
The “TypeError: a bytes-like object is required, not ‘str'” error occurs when a function expects a bytes-like object but is provided with a regular string. By understanding the difference between strings and bytes and by using the conversion methods described in this article, you can resolve this error and improve the efficiency and accuracy of your Python code.
Are you interested in learning more about Python errors and how to resolve them?