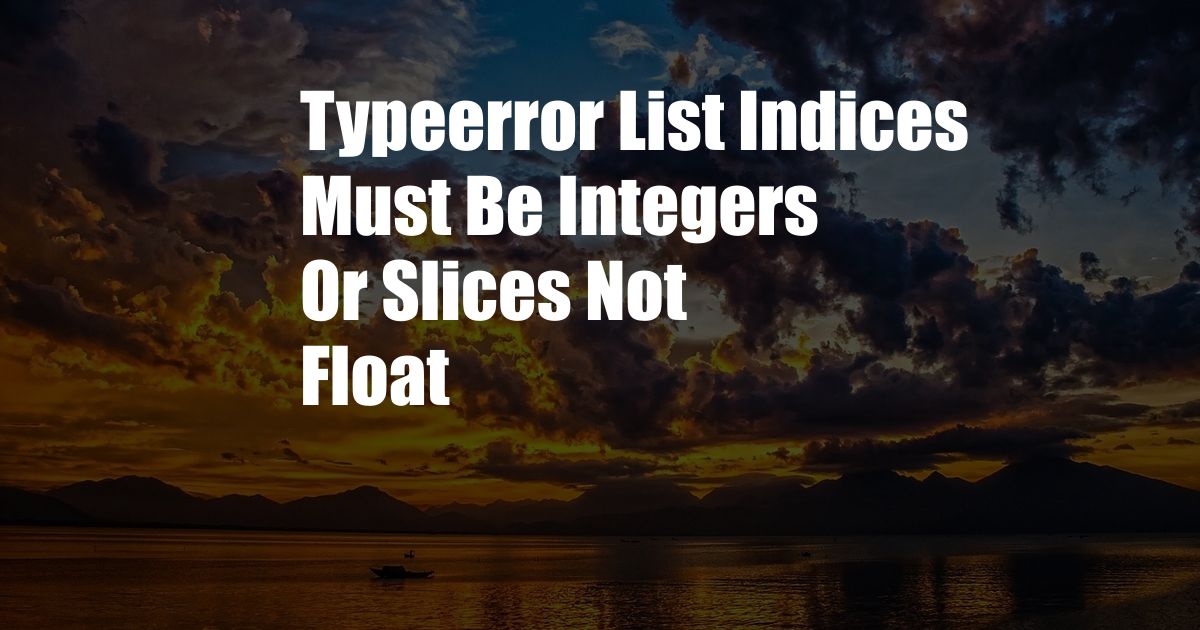
TypeError: list indices must be integers or slices, not float
Have you ever spent hours trying to debug a Python error that makes absolutely no sense? Well, I recently encountered the infamous “TypeError: list indices must be integers or slices, not float” error, and boy, was it a head-scratcher.
I was working on a data science project, happily iterating through a list of numbers and performing some calculations. However, out of the blue, my code crashed with this cryptic error. I knew that list indices should be integers, but I couldn’t fathom why I was getting a float as an index. After much head-banging and debugging, I finally realized the culprit: I had accidentally divided a list index by another number, resulting in a floating-point number.
What is a TypeError?
A TypeError in Python is raised when an operation or function is applied to an object of an inappropriate type. For instance, trying to add a string to an integer will result in a TypeError. In our case, attempting to use a float as a list index violates Python’s strict type system, leading to the error “TypeError: list indices must be integers or slices, not float”.
To avoid this error, we must ensure that list indices are always integers or slices. If you need to work with floating-point indices, consider converting them to integers using the built-in int()
function.
How to fix the error
There are several ways to fix this error, depending on the logic of your code and the desired behavior.
- Convert the float to an integer: If you need to use a floating-point index, convert it to an integer using the
int()
function. For example, if you have a list called `my_list`, you can access the element at index 3.5 by converting it to `int(3.5)`. - Use slicing: Slicing allows you to access a range of elements in a list using a colon (
:
). If you need to access elements beyond the end of the list, you can use a negative slice. For instance, `my_list[-1]` accesses the last element of `my_list`. - Check the type of the index: Before using a variable as a list index, check its type using the
type()
function. If it’s not an integer or a slice, raise an exception or convert it to the appropriate type.
Conclusion
Understanding and resolving the “TypeError: list indices must be integers or slices, not float” error is essential for any Python programmer. By following the tips and advice outlined above, you can effectively avoid this error and write more robust and reliable code. Remember, error messages in Python are valuable clues to help you identify and rectify issues in your code. So, take the time to understand the error message and find the root cause of the problem.
Are you facing any issues related to this error or have any additional insights? I would love to hear your thoughts and experiences in the comments below.