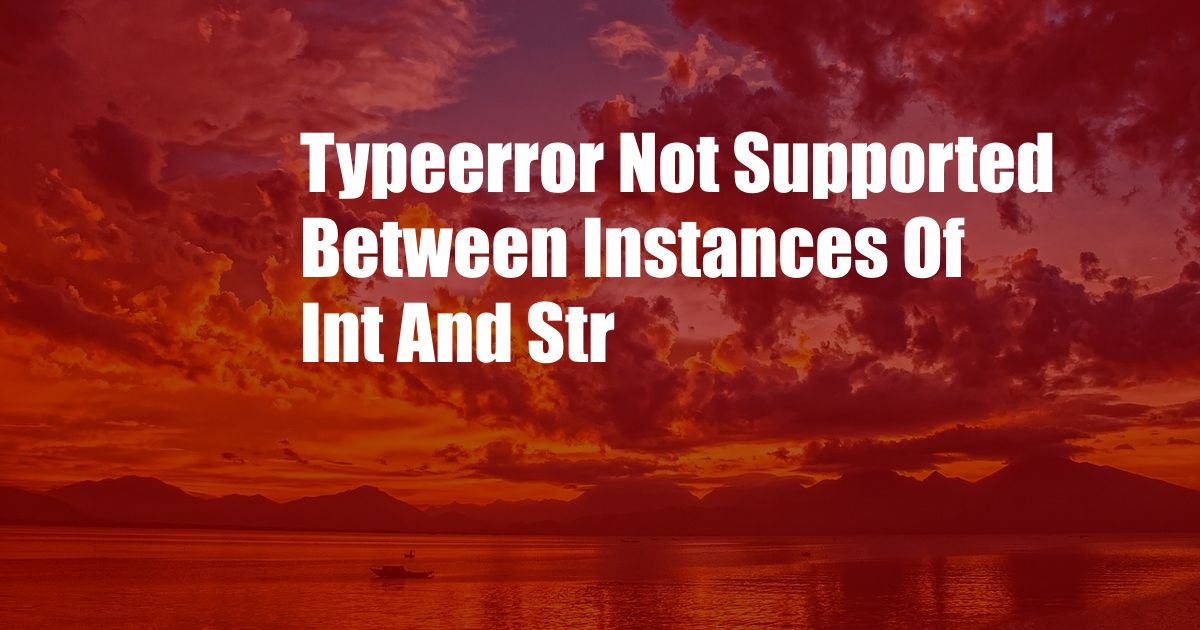
TypeError: Not Supported Between Instances of Int and Str
While delving into the enigmatic world of coding, I encountered a perplexing error message that halted my progress: “TypeError: not supported between instances of ‘int’ and ‘str’.” Like a cryptic puzzle, it beckoned me to unravel its enigmatic depths.
Embarking on a quest for enlightenment, I meticulously combed through documentation, consulted fellow coders, and immersed myself in online forums. Through diligent research, I unraveled the intricacies of this enigmatic error.
Operand Mismatch: A Tale of Two Data Types
At the heart of the “TypeError: not supported between instances of ‘int’ and ‘str'” lies a fundamental mismatch between data types. In programming, variables can hold different types of data, such as integers (‘int’), strings (‘str’), or booleans. Each data type possesses unique characteristics and rules that govern how they can be combined and manipulated.
In this case, the error arises when an operation is attempted between an integer and a string. For instance, let’s consider the following Python code:
>>> x = 10
>>> y = "Hello"
>>> x + y
TypeError: unsupported operand type(s) for +: 'int' and 'str'
As you can observe, the ‘+’ operator is typically used to add two integers. However, when one of the operands is a string, Python raises the “TypeError: not supported between instances of ‘int’ and ‘str'” error. This is because the ‘+’ operator is not defined for the combination of an integer and a string.
Understanding Data Type Compatibility
To avoid such errors, it is crucial to understand data type compatibility. Different programming languages have their own set of rules that determine which operations are supported between different data types. In Python, for instance, the ‘+’ operator can only be used to add two integers or two strings.
To perform operations between different data types, you may need to employ type conversion functions. For example, to add an integer to a string, you can convert the integer to a string using the ‘str()’ function:
>>> x = 10
>>> y = "Hello"
>>> z = str(x) + y
>>> print(z)
Hello10
By converting the integer ‘x’ to a string, we can successfully concatenate it with the string ‘y’.
Preventing “TypeError: Not Supported Between Instances of Int and Str”
To prevent encountering this error, it is advisable to follow these tips:
- Use type checking to ensure that operands have compatible data types.
- Employ type conversion functions when necessary to convert data types.
- Refer to language documentation to understand the supported operations for different data types.
These precautions will help you avoid common pitfalls and write more robust and error-free code.
FAQ on TypeError: Not Supported Between Instances of Int and Str
Q: Why does Python raise a “TypeError: not supported between instances of ‘int’ and ‘str'” error?
A: Python raises this error when an operation is attempted between an integer and a string. This is because the ‘+’ operator is not defined for the combination of an integer and a string.
Q: How can I prevent this error?
A: To prevent this error, use type checking to ensure that operands have compatible data types. If necessary, employ type conversion functions to convert data types.
Q: What is type conversion?
A: Type conversion is the process of converting a variable from one data type to another. In Python, you can use functions like ‘int()’, ‘str()’, and ‘float()’ to convert between different data types.
Conclusion
The “TypeError: not supported between instances of ‘int’ and ‘str'” error serves as a reminder of the importance of understanding data type compatibility in programming. By adhering to data type rules, employing type checking, and utilizing type conversion functions, you can effectively avoid this error and write more robust and efficient code.
If you are interested in delving deeper into the world of data types and error handling, I highly recommend exploring the official Python documentation and engaging with online coding communities for further insights and support.