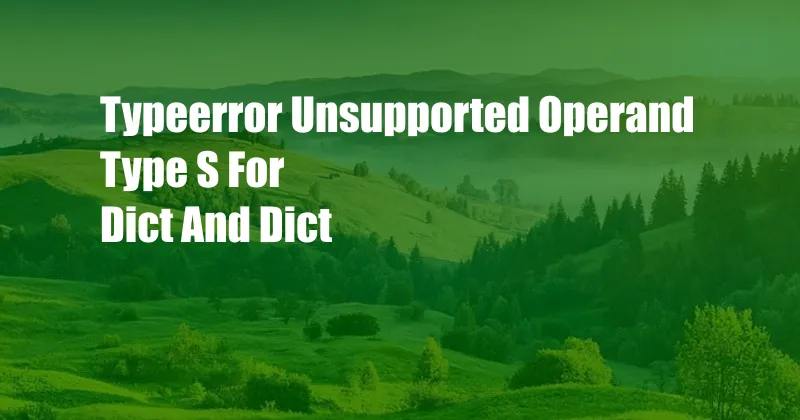
TypeError: unsupported operand type(s) for dict and dict
As an avid data enthusiast, I’ve grappled with my fair share of Pythonic puzzles. One particularly perplexing error that often leaves me scratching my head is TypeError: unsupported operand type(s) for dict and dict. This exception arises when attempting to perform operations on dictionaries in ways that are not supported by Python. To unravel the mystery behind this error, let’s delve into the world of dictionaries and uncover the reasons why this exception occurs.
Dictionaries are Python’s built-in data structure to represent key-value pairs. Each key is uniquely associated with a corresponding value, providing a convenient way to store and retrieve data. However, there are certain constraints on the operations that can be performed on dictionaries. One such limitation is the inability to perform arithmetic operations (such as addition, subtraction, multiplication, division, and modulo) directly between two dictionaries. Attempting to do so will result in the TypeError: unsupported operand type(s) for dict and dict exception.
Understanding the Exception
To comprehend the rationale behind this exception, we need to understand that dictionaries are mutable data structures. Operations like addition or subtraction would require modifying the existing dictionaries, which is not a valid operation in Python. Dictionaries are designed to maintain key-value relationships, not to perform arithmetic computations.
The purpose of dictionaries is to provide a mapping between keys and values. Mixing dictionaries with arithmetic operations could lead to ambiguity and undefined behavior. For example, what would it mean to add two dictionaries together? Should it combine the keys or the values? How would it handle duplicate keys? To avoid such confusion and ensure consistency, Python prohibits arithmetic operations directly on dictionaries.
Alternative Approaches
While dictionaries themselves do not support arithmetic operations, there are alternative ways to achieve similar functionality. If your goal is to combine data from multiple dictionaries, you can use the update() method or create a new dictionary that merges the desired keys and values. For example:
# Combining dictionaries using the update() method
dict1 = 'a': 1, 'b': 2
dict2 = 'c': 3, 'd': 4
dict1.update(dict2)
print(dict1) # Output: 'a': 1, 'b': 2, 'c': 3, 'd': 4
# Creating a new dictionary to merge keys and values
dict3 = **dict1, **dict2
print(dict3) # Output: 'a': 1, 'b': 2, 'c': 3, 'd': 4
Alternatively, if you want to perform arithmetic operations on the values stored in dictionaries, you can access the values individually and then perform the desired calculations:
dict1 = 'a': 1, 'b': 2
dict2 = 'c': 3, 'd': 4
# Adding the values of 'a' and 'c'
sum_of_values = dict1['a'] + dict2['c']
print(sum_of_values) # Output: 4
Conclusion
TypeError: unsupported operand type(s) for dict and dict is an exception that occurs when attempting to perform arithmetic operations directly between two dictionaries. This is because dictionaries are designed to maintain key-value relationships, not to perform mathematical computations. However, there are alternative ways to combine data from multiple dictionaries or perform operations on the values stored in dictionaries. By understanding the limitations and employing the appropriate techniques, you can work around this exception and effectively utilize dictionaries in your Python programs.
Are you intrigued by the world of data structures and eager to dive deeper into the nuances of Python dictionaries? If so, I encourage you to explore additional resources and continue experimenting with different approaches to data manipulation. Python’s versatility and extensive libraries provide endless opportunities for growth and discovery.
FAQs
Q: Can I add two dictionaries together in Python?
A: While you cannot perform direct addition of two dictionaries, you can use the update() method to merge the dictionaries or create a new dictionary by combining the keys and values using the ** operator.
Q: How can I perform arithmetic operations on the values stored in dictionaries?
A: Access the values individually using the dictionary keys and then perform the desired calculations.
Q: What is the purpose of dictionaries in Python?
A: Dictionaries provide a mapping between keys and values, allowing for efficient storage and retrieval of data.
Q: Why are arithmetic operations not supported on dictionaries in Python?
A: Dictionaries are designed to maintain key-value relationships, and arithmetic operations on dictionaries could lead to ambiguity and undefined behavior.