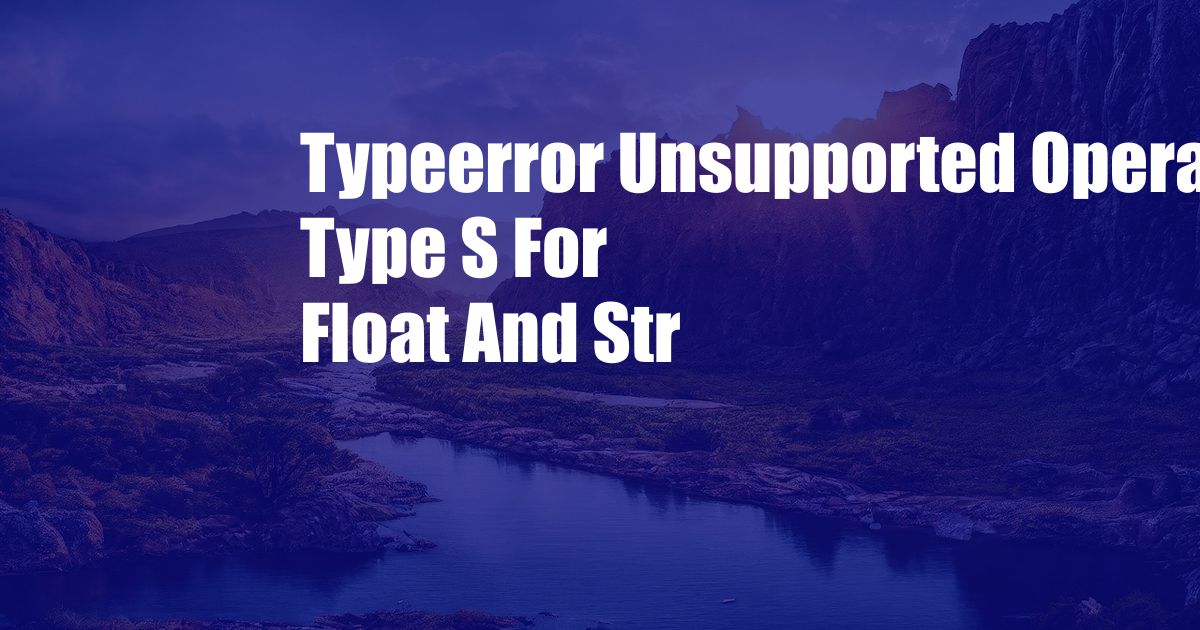
TypeError: Unsupported Operand Type(s) for Float and Str in JavaScript
Have you ever encountered a perplexing error message in your JavaScript code that reads, “TypeError: Unsupported operand type(s) for +: ‘float’ and ‘string’?” If so, you’re not alone. This error arises when you attempt to perform an arithmetic operation, such as addition or subtraction, on operands of incompatible types. While this error may seem obscure, understanding its cause and how to resolve it can significantly improve your coding skills. In this comprehensive guide, we will delve into the depths of this error, exploring its origins, implications, and practical solutions.
At its core, the “TypeError: Unsupported operand type(s) for +” error occurs when JavaScript encounters an attempt to perform an arithmetic operation on operands that are not both numbers. JavaScript is a dynamically typed language, meaning that it does not enforce strict type checking during code execution. Consequently, if you attempt to add a string to a number, JavaScript will attempt to coerce the string into a number and perform the addition. However, this coercion can lead to unexpected results and potential errors. To avoid this pitfall, it’s crucial to ensure that both operands involved in arithmetic operations are of the same type, typically numbers.
Operand Type Coercion in JavaScript
JavaScript’s dynamic typing allows for implicit type coercion, which means that JavaScript can automatically convert values from one type to another. While this flexibility can be useful in certain scenarios, it can also lead to errors if not handled carefully. When performing arithmetic operations, JavaScript follows specific coercion rules to convert non-numeric operands into numbers.
For instance, if you attempt to add the string “5” to the number 10, JavaScript will coerce the string “5” into the number 5 and perform the addition, resulting in the expected sum of 15. However, if you attempt to add the string “abc” to the number 10, JavaScript will fail to coerce the string into a number, resulting in the “TypeError: Unsupported operand type(s) for +” error.
Preventing the TypeError: Unsupported Operand Type(s) for Float and Str
To effectively prevent the occurrence of the “TypeError: Unsupported operand type(s) for +” error, it’s essential to explicitly check the types of operands before performing arithmetic operations. This can be achieved using the typeof operator, which returns the type of a variable. By incorporating type checking into your code, you can ensure that only compatible operands are involved in arithmetic operations, thereby eliminating the potential for errors.
For example, the following code snippet demonstrates how to use type checking to prevent the error:
const num1 = 10;
const str1 = "5";
if (typeof num1 === 'number' && typeof str1 === 'number')
const result = num1 + str1;
console.log(result); // Outputs: 15
else
console.log("Error: Unsupported operand types for addition.");
Additional Tips for Avoiding the Error
In addition to using explicit type checking, there are several other best practices that can help you avoid the “TypeError: Unsupported operand type(s) for +” error:
- Always ensure that variables used in arithmetic operations are initialized with appropriate values.
- Use strict mode in your code, which enforces stricter type checking and helps identify potential errors early on.
- Consider using a linter or type checker to identify potential errors and enforce coding standards.
FAQs on TypeError: Unsupported Operand Type(s) for Float and Str
Q: What causes the “TypeError: Unsupported operand type(s) for +” error?
A: This error occurs when JavaScript encounters an attempt to perform an arithmetic operation on operands that are not both numbers.
Q: How can I prevent the “TypeError: Unsupported operand type(s) for +” error?
A: You can prevent this error by explicitly checking the types of operands before performing arithmetic operations using the typeof operator.
Q: Is there a way to handle the “TypeError: Unsupported operand type(s) for +” error gracefully?
A: Yes, you can use try…catch blocks to handle this error gracefully and provide informative error messages to users.
Conclusion
Understanding and resolving the “TypeError: Unsupported operand type(s) for +” error is essential for writing robust and reliable JavaScript code. By comprehending the underlying causes of this error, implementing type checking, and adhering to best practices, you can effectively prevent this error from disrupting your code and ensure seamless operation.
If you have found this article informative and helpful, please consider sharing it with others who may encounter similar challenges. Your feedback and engagement are invaluable in our mission to provide accessible and high-quality technical content. Thank you for reading!